I was having trouble with the hardhat bytecode plugin so I wrote a little nodejs script which will print off the size of my compiled Solidity contracts. You’ll need nodejs installed and can then run it like this.
npm install fs
npm install path
npm install child_process
npx hardhat compile
node ./analyze_bytecode.js
You should get an ouput like this:
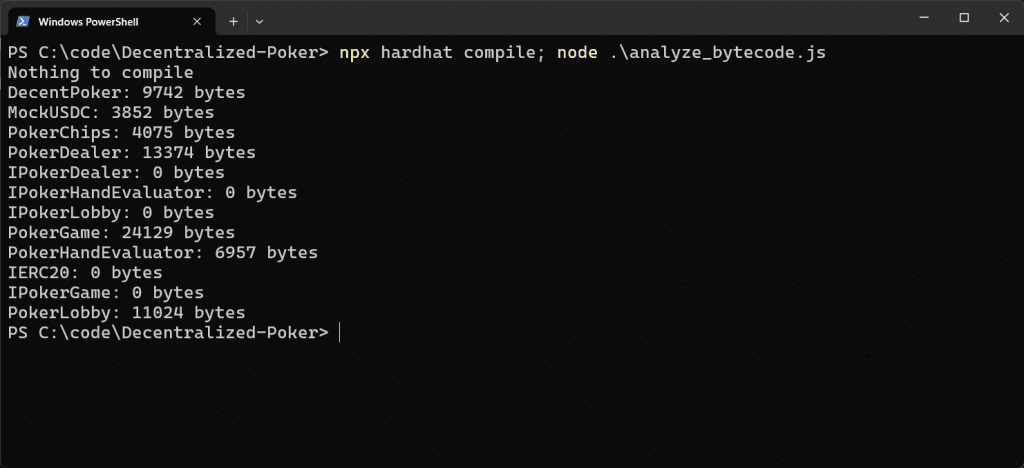
Here’s the code to save to analyze_bytecode.js file in the root of your repository.
const fs = require('fs');
const path = require('path');
const { exec } = require('child_process');
async function main() {
const artifactsPath = path.join(__dirname, 'artifacts', 'contracts');
// Function to recursively read files from a directory
const readFiles = dir =>
fs.readdirSync(dir).reduce((files, file) =>
fs.statSync(path.join(dir, file)).isDirectory() ?
files.concat(readFiles(path.join(dir, file))) :
files.concat(path.join(dir, file)), []);
// Get all .json files from the artifacts/contracts directory
const files = readFiles(artifactsPath).filter(file => file.endsWith('.json'));
for (const file of files) {
const content = JSON.parse(fs.readFileSync(file, 'utf8'));
if (content.deployedBytecode) {
const bytecode = content.deployedBytecode;
const bytecodeSize = bytecode.length / 2 - 1; // Each byte is represented by 2 hex characters
console.log(`${path.basename(file, '.json')}: ${bytecodeSize} bytes`);
}
}
}
main().catch(console.error);