One powerful feature in Rust is how it organizes code into modules and allows the reuse of code with structs and methods. In this tutorial, we’ll walk through a simple example to illustrate how to define and use a struct in Rust and how to import modules for organization.
Step 1: Define the Module and Struct
In Rust, modules help organize your code into logical units. You can group related code together and make it reusable. Let’s start by defining a module with a struct and some methods.
File: mymodule.rs
pub struct User {
pub name: String,
pub age: u32,
}
impl User {
pub fn new(name: String, age: u32) -> User {
User { name, age }
}
pub fn greet(&self) -> String {
format!("Hello {}, you wish you were {}!", self.name, self.age)
}
}
Step 2: Use the Module in main.rs
Now that we have a module, we can use it in our main.rs
file by importing it. In Rust, mod
is used to include external module files.
File: main.rs
mod mymodule;
fn main() {
let user = mymodule::User::new(String::from("James"), 21);
println!("{}", user.greet());
}
Step 3: Running the Code
After writing these two files (mymodule.rs
and main.rs
), you can compile and run your program by navigating to the project directory and running:
cargo run
Finished `dev` profile [unoptimized + debuginfo] target(s) in 0.00s
Running `target\debug\rstest.exe`Hello James, you wish you were 21!
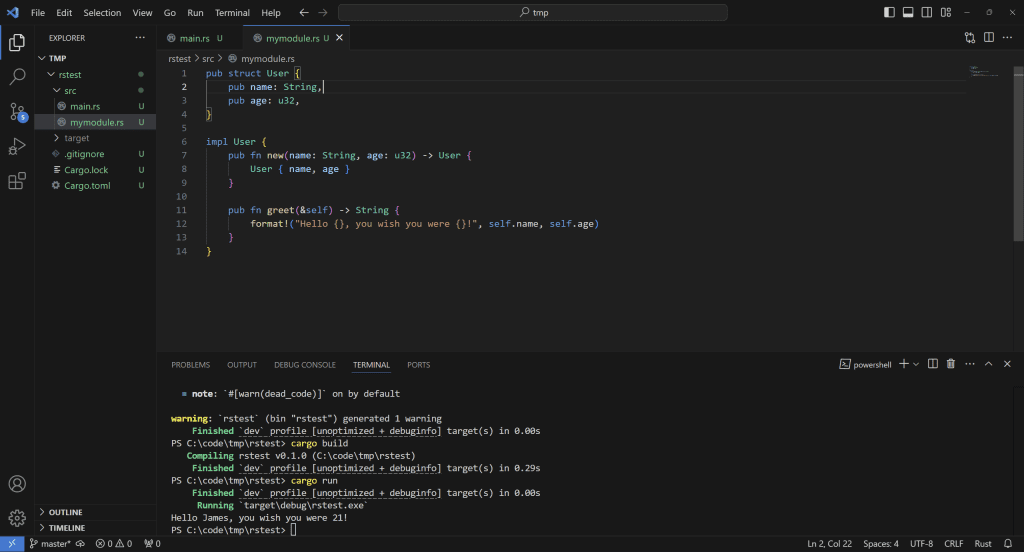
How It Works
The User
struct in mymodule.rs defines a simple data structure that holds two fields: name
, which is a String, and age
, which is an unsigned 32 bit integer (u32). Structs in Rust are used to group related data together, allowing you to create instances of that data and manage it through methods. In this example, the User
struct models a user’s basic information, which can be easily accessed or manipulated via methods.
Rust modules, like the mymodule.rs in this example, are used to organize code into separate files. This approach helps keep the codebase clean and manageable, especially in larger projects. By placing the User
struct inside the mymodule.rs file and importing it into main.rs using the mod mymodule; statement, we can use the struct and its methods from outside the module. This modular design improves code organization and allows for reusability in different parts of the project.
Methods are implemented for the User
struct to interact with its data. The new
method is a constructor that initializes a User
instance with the given name
and age
. The greet method formats and returns a greeting message based on the user’s name and age. These methods provide a simple interface for interacting with the struct’s data, keeping the code both concise and expressive.
This simple example shows how to create a Rust module, define a struct with methods, and import it into another file. Modules in Rust provide a clean way to organize and reuse code, and structs allow you to represent data with associated methods to manipulate that data. By following this pattern, you can keep your code modular and maintainable.