I get a lot of comments from Solidity tutorial videos and in Github issues asking how to resolve common solidity error codes. In this article I’ll go through some of the most frequent solidity issues, what they mean and how to resolve them.
UNPREDICTABLE_GAS_LIMIT
This is the most common and confusing error in Solidity
- ‘UNPREDICTABLE_GAS_LIMIT’ reason: cannot estimate gas
- cannot estimate gas; transaction may fail or may require manual gas limit
The error doesn’t actually relate to gas or paying transaction fees which is the most confusing and frustrating thing in Solidity.
The error is popped whenever a transaction will fail. So if you have a wrong contract address or are doing something stupid you’ll get this generic gas error.
Most common causes I see are related to moving contracts from testnets to mainnets. The token contract addresses will often change or there wont be a version of Uniswap on their local testnet which causes the deployment or transaction to fail.
Another absolute classic is copying the contract address from the URL in etherscan which converts it to all lowercase, which then fails the checksum, resulting in a gas error which is about as useful as a chocolate tea pot. You’ll often see gas limit and errors like this:
Error: invalid address (argument=”address”, value=””, code=INVALID_ARGUMENT
If you are getting the upredicatable_gas_limit error and have checked the stupid stuff then I’d recommend running it on a public testnet and putting the transaction hash into Tenderly to debug it from there.
TRANSFER_FROM_FAILED
The error ‘TransferHelper: TRANSFER_FROM_FAILED’ or safeTransferFrom compile error is related to the contract function not being able to move tokens on your behalf. Somewhere in the code there will be a transferFrom() or safeTransferFrom() function which will attempt to move tokens from a 3rd party account.
The most common cause for this error is not calling token.approve() prior to calling transferFrom()
Note that you need to call approve() from the holders wallet. I’ve got stuck many times trying to transferFrom the contract address or approving the wrong account. Use msg.sender
for the user address calling the function and address(this)
for the contract.
The common approach is to approve the transaction first as a separate transaction from the users wallet, then the contract will call transferFrom(msg.sender, receiverAddress, amount); which will send the tokens from the users wallet that called the function.
This can also go wrong when devs get confused on the frontend between ETH and WEI. Both these functions take a WEI value including the decimals. Note that most but not all tokens have 18 decimals and USDC is the common exception with 6. If you approve 1 and then try to transferFrom 1000000000000000000 it wont work and you’ll get the a TRANSFER_FROM_FAILED error.
ADDRESS PAYABLE
The TypeError: “send” and “transfer” are only available for objects of type “address payable”, not “address” error occurs when we try to send ETH from an address without declaring it payable.
This is a simple fix where we just need to encapsulate the address in a payable wrapper before calling transfer.
payable(someAddress).transfer(address(this).balance);
If you get an error along the lines of:
Note: The called function should be payable if you send value and the value you send should be less than your current balance.
Then you may also need to add payable to the function modifiers so it can receive ETH.
WRONG ARGUMENT COUNT
This is something you’ll see if you forget to pass a constructor argument to a contract when deploying or call a function with the wrong amount of arguments.
Error (6160): Wrong argument count for function call: 0 arguments given but expected 1
Check the inputs and anything on the frontend which might have caused the wrong number of function inputs to be sent with the function call.
ERROR ENCODING ARGUMENTS
This is a type error and usually comes from trying to send variables from a loosely typed language in Javascript to a strongly typed language in Solidity.
The most common one is
Error encoding arguments: Error: invalid BigNumber string
The BigNumber library takes inputs as a string because Maths in Javascript is hit and miss. Try doing 0.8 - 0.1
in a browser console window

The error relates to being unable to convert between types or missing a function input or getting the function inputs the wrong way around.
RPC ERROR CODES
EIP1474 sets out standardised error codes for RPC nodes and Solidity devs and web3 frontend devs will often come across these.
Code | Message | Meaning |
---|---|---|
-32700 | Parse error | Invalid JSON |
-32600 | Invalid request | JSON is not a valid request object |
-32601 | Method not found | Method does not exist |
-32602 | Invalid params | Invalid method parameters |
-32603 | Internal error | Internal JSON-RPC error |
-32000 | Invalid input | Missing or invalid parameters |
-32001 | Resource not found | Requested resource not found |
-32002 | Resource unavailable | Requested resource not available |
-32003 | Transaction rejected | Transaction creation failed |
-32004 | Method not supported | Method is not implemented |
-32005 | Limit exceeded | Request exceeds defined limit |
-32006 | JSON-RPC version not supported | Version of JSON-RPC protocol is not supported |
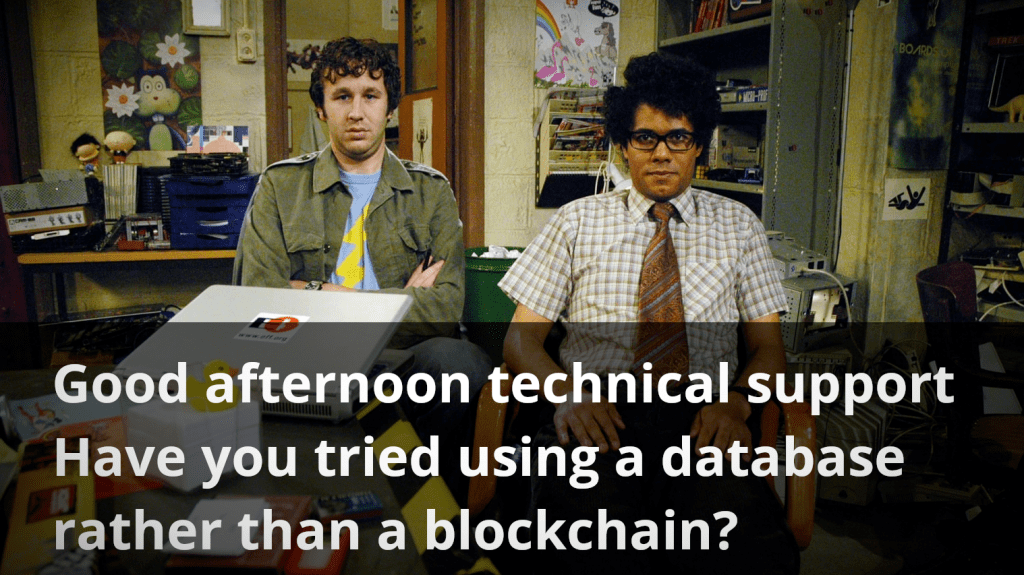