To check a wallet token balance on the Ethereum blockchain using Python you will need the following:
- Infura API Key You can get this by signing up at Infura.
- Python Installed on your device, download here.
- Web3.py This is a Python library for interacting with the Ethereum blockchain.
- ENS.py This is a Python library for resolving ENS names.
Once we have our API key ready and Python installed we can install web3.py using the following command
pip install web3 ens
Now let’s create a file called enslookup.py and add the following code. Enter your Infura API key on line 5. Code is also available in the Github repository: https://github.com/jamesbachini/Web3-Python-Scripts
import argparse
from web3 import Web3
infura_url = "https://mainnet.infura.io/v3/YOUR_API_KEY_HERE"
web3 = Web3(Web3.HTTPProvider(infura_url))
parser = argparse.ArgumentParser(description="Look up an Ethereum Name Service (ENS) name.")
parser.add_argument("name", type=str, help="The ENS name to look up, e.g., james.eth")
args = parser.parse_args()
address = web3.ens.address(args.name)
if address:
print(f"Address found for {args.name} - {address}")
else:
print(f"ENS name {args.name} could not be found")
Now lets run the script and give it a name to lookup as the first argument
python ./enslookup.py james.eth
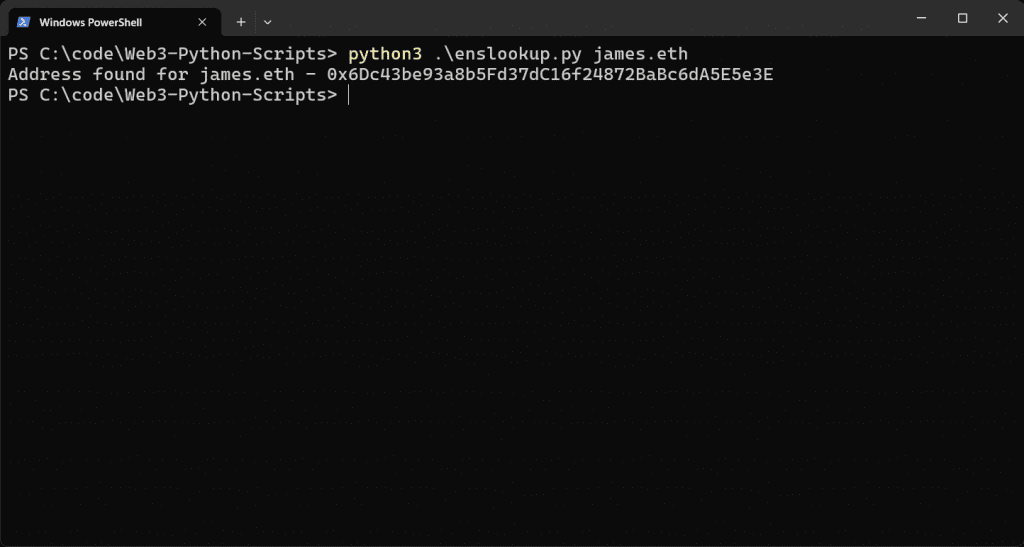