In this tutorial we will be writing some code in Rust, compiling to web assembly (WASM) and then running that code in a browser. This enables high performance applications to be run within a web dev setting.
In this tutorial I’ll be using windows subsystem for Linux. Let’s first install Rust and wasm-pack (more instructions for how to install rust on different operating systems here)
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
cargo install wasm-pack
Now let’s set up a new directory and add the WebAssembly target
cargo new hello-wasm --lib
cd hello-wasm
rustup target add wasm32-unknown-unknown
We will then go into the directory and open up the Cargo.toml file and add the following dependency and library
[dependencies]
wasm-bindgen = "0.2"
[lib]
crate-type = ["cdylib"]
Now go in and edit src/lib.rs, replace the entire file with the code below
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
pub fn loop(name: &str) -> String {
format!("Hello, {}!", name)
}
Let’s build and pack it into a wasm file. This command compiles the Rust code to WASM and places the output in the pkg directory.
wasm-pack build --target web
Now let’s embed this code into a web page called index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple WASM Example</title>
</head>
<body>
<h1>Simple Rust + WASM Example</h1>
<input type="text" id="name-input" placeholder="Enter your name" />
<button id="greet-btn">Greet</button>
<h2 id="greeting"></h2>
<script type="module">
import init, { greet } from './pkg/hello_wasm.js';
async function run() {
await init(); // Initialize the WASM module
const button = document.getElementById('greet-btn');
const input = document.getElementById('name-input');
const greeting = document.getElementById('greeting');
button.addEventListener('click', () => {
const name = input.value;
greeting.textContent = greet(name);
});
}
run();
</script>
</body>
</html>
We can pop this onto a webserver with the wasm files in pkg/ and run it from a browser.
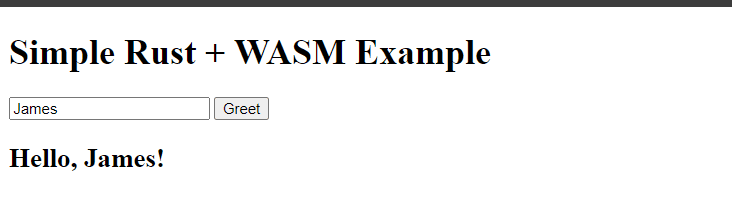
This is a basic example of how to get data from javascript in a web browser to a wasm module executing web assembly built from Rust code.