A callback function in Solidity allows us to pass a function reference from one contract to another and have it execute as part of an atomic transaction.
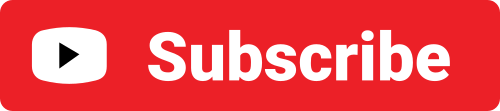
Let’s first take a look at an example from the Solidity Snippets Github repo. Full code at: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Callback.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
contract Parent {
uint public parentVariable;
function parentFunc(uint _parentVariable, function(uint) external _callback) public {
parentVariable = _parentVariable;
_callback(_parentVariable * 2);
}
}
contract Child {
address public parentAddress;
uint public childVariable;
constructor(address _parentAddress) {
parentAddress = _parentAddress;
}
function run(uint _newVar) public {
Parent(parentAddress).parentFunc(_newVar, this.callback);
}
function callback(uint _childVariable) external {
childVariable = _childVariable * 2;
}
}
This code is an example of how contracts can interact with each other using callbacks. The run
function of the Child contract calls the parentFunc() function of the Parent
contract, and the parentFunc() function in turn calls the callback() function of the Child contract.
When the run() function of the Child contract is called, it invokes the parentFunc function of the Parent contract and passes it the _newVar parameter along with a reference to the callback() function of the Child contract. The parentFunc() function of the Parent contract is executed, it sets the value of parentVariable to what we define and then calls the callback function passed to it, passing in _parentVariable multiplied by 2 as an argument. When the callback function of the Child contract is called, it sets the value of childVariable to _childVariable multiplied by 2 again so eventually:
childVariable == parentVariable * 4;
Callback functions are used widely in things like flash loans where you take out a loan and once those funds have been transferred it calls your doWhileIHaveTheLoan() function and then once that has executed it checks to see you have paid it back and reverts if you haven’t.#
I hope this article has provided an introduction and useful code example for how you can use callbacks in Solidity to move data between smart contracts.
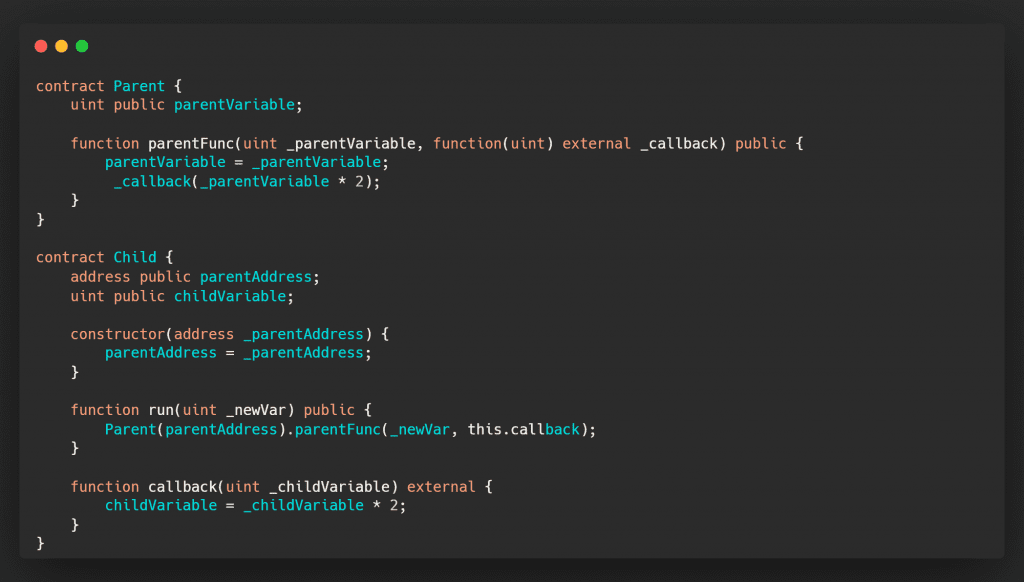