Never have I sounded more like an Ethereum maxi, but there is a point beyond the provocative title. In this post I’m going to explore the potential for investors to use carbon credit tokens on Ethereum to offset the electrical consumption of BTC mining on their Bitcoin holdings.
This novel idea provides the opportunity to dive into some Solidity code and look at how a carbon offsetting system might work.
Carbon Offsetting Solidity Code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract CarbonCreditToken is ERC20 {
address constant dai = 0x6B175474E89094C44Da98b954EedeAC495271d0F;
address constant carbonFund = address(0x0);
uint offsetCostPerKG = 7e15; // $0.007 USD
// Estimated carbon usage per annum of BTC Network
// https://ccaf.io/cbnsi/cbeci/ghg
uint public networkCarbonUsage = 75_000_000_000;
uint public carbonUsagePerBTC = networkCarbonUsage / 20_000_000;
constructor() ERC20("CarbonCreditToken", "CCT") {}
// Calculate carbon offset requirement for a given number of Bitcoin
function calculateOffsetCost(uint _numBitcoin) public view returns (uint) {
return _numBitcoin * carbonUsagePerBTC * offsetCostPerKG;
}
// Convenience function for calculating the number of credits to buy and buying them
function offsetCarbonFootprint(uint _numBitcoin) external payable {
uint cost = calculateOffsetCost(_numBitcoin);
IERC20(dai).transferFrom(msg.sender, carbonFund, cost);
_mint(msg.sender, _numBitcoin * 10 ** decimals());
}
function burn(uint256 _amount) public {
_burn(msg.sender, _amount);
}
}
How The Carbon Contract Works
The contract uses the standard OpenZeppelin ERC20 library to create a token which equates to offsetting the carbon cost of 1 BTC per year.
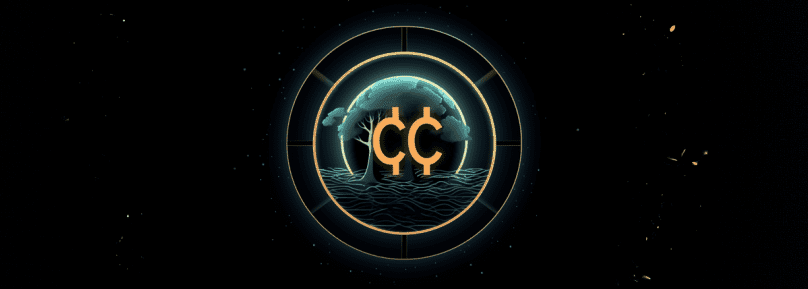
- We start by setting up some addresses for the dai stablecoin and a carbon fund account which could be a traditional organisation or a DAO wallet.
- We then set some assumptions for
offsetCostPerKG
,networkCarbonUsage
&carbonUsagePerBTC
These give the estimated carbon usage of the Bitcoin network and the carbon usage per individual BTC. This data is based on some research out of Cambridge Uni. I’ve left them as fixed constants for simplicity but in a production contract you would probably want the option to update them based on latest data. - The calculateOffsetCost() function takes as input the number of Bitcoins a user owns and then calculates the cost in DAI to offset the carbon footprint for that amount.
- The offsetCarbonFootprint() function uses the cost from the previous function, transfers that amount of DAI from the user to the carbonFund, and then mints CarbonCreditTokens for the user proportional to the number of Bitcoins for which they offset the carbon footprint. The input takes the whole number of Bitcoin but again in a production environment it might be better to use satoshis. This contract assumes that the user has already approved the contract to spend the required DAI on their behalf before the function is called.
- The final burn() function allows a user to burn the specified amount of their CarbonCreditTokens at the end of the year term.
Code is untested and for demonstration purposes only, not suitable to be deployed anywhere near mainnet.
For those wondering, based on these rough and under-researched calculations the cost to offset 1 Bitcoin would be $262.50 USD per year