Conditional statements & loops in Solidity are fairly intuitive and follow the conventions of other languages such as Javascript. In this article we will go through some examples of each before looking at the relational operators we can use within these statements.
if statement
The “if” statement is used to execute a block of code only if a certain condition is true. We can create further logic queries using “else if” and “else” to control the flow through the contract.
uint number = 7;
if (number < 5) {
// doesn't run
} else if (number == 7) {
// only this runs
} else {
// doesn't run
}
// we can also do one liners
if (number == 7) number = 8;
switch statement
Switch statements offer a different pattern for similar functionality to an if statement. In a switch statement, the value of the variable is compared to the values specified in each “case” statement. If a match is found, the corresponding block of code is executed. If no match is found, the code in the “default” block is executed. The “break” keyword is used to exit the switch statement after a block of code is executed.
switch (variable) {
case value1:
// Code to execute if the value of variable is value1
break;
case value2:
// Code to execute if the value of variable is value2
break;
default:
// Code to execute if the value of variable is neither value1 nor value2
break;
}
for loop
In a for loop, the “initialization” statement is executed once at the beginning, the “condition” is checked before each iteration, and the “increment/decrement” statement is executed at the end of each iteration.
for (uint i = 0; i < 100; i++) {
if (i == 25) continue; // skip to next iteration
if (i == 50) break; // exit loop
}
This is often used to iterate over arrays and avoid repeatable code. Be careful with using for loops over dynamic arrays because if the array grows too large it can exceed the max gas cost for a transaction creating a denial of service attack.
address[] users = [0x0,0x0];
for (uint i = 0; i < users.length; i++) {
// watch out for unbounded loops
}
while loop
The while loop is used when we do not know the number of times we want to execute a block of code, but we do know the condition under which the loop should continue. The code inside the loop is executed repeatedly if and as long as the “condition” is true.
uint x = 0;
while (x < 5) {
x ++;
// this will run five times x = 1-5
}
do-while loop
Do-while loop is similar to a while loop, but the code inside the loop is executed at least once, regardless of whether the condition is true or false. The code inside the loop is executed once before the “condition” is checked. If the condition is true, the code is executed repeatedly until the condition is false.
uint x = 0;
do {
x ++;
// runs at least once
} while (x < 5);
Relational Operators
Here is a list of the different relational operators we can use within these statements and loops.
==
(equal to): checks if two values are equal.!=
(not equal to): checks if two values are not equal.<
(less than): checks if the left operand is less than the right operand.>
(greater than): checks if the left operand is greater than the right operand.<=
(less than or equal to): checks if the left operand is less than or equal to the right operand.>=
(greater than or equal to): checks if the left operand is greater than or equal to the right operand.
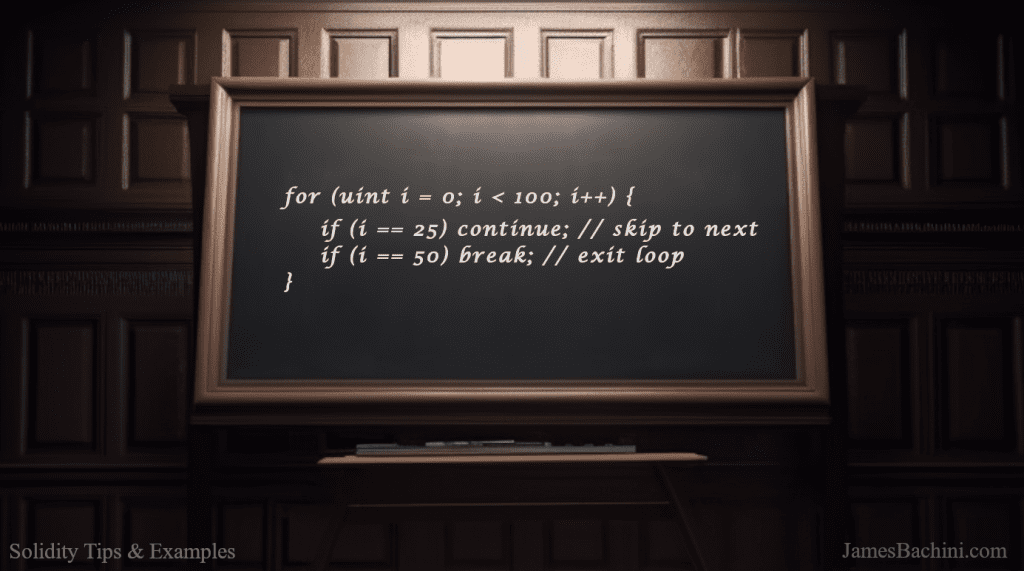
These patterns form the constructional building blocks that Solidity developers use to create their smart contracts. Conditional statements allow developers to execute a block of code only when a certain condition is true, loops enable them to repeat a block of code until a certain condition is false.
By using conditional statements and loops effectively, developers can create smart contracts that execute their intended logic in a decentralized and trustless manner.