ERC20 tokens have become the de facto standard for creating tokens on Ethereum and are widely used in many DeFi protocols for things like governance and utility tokens.
- Why Use ERC20 Token
- Simple ERC20 Token Contract
- The OpenZeppelin Library
- ERC20 Token Functions
- Conclusion
Why Use ERC20 Token
Solidity developers use ERC20 tokens because they are the industry standard for fungible tokens (where every token is equal to another one). The ERC20 token standard offers:
- Interoperability – ERC20 tokens are interoperable with other ERC20 tokens and can be easily integrated with other decentralized applications, such as exchanges and wallets.
- Standardization – The ERC20 token standard provides a clear and well-defined interface for creating and managing tokens. This makes it easier for developers to create and maintain their own tokens, as they can rely on a consistent set of rules and functions.
- Decentralization – As standard ERC20 tokens are fully decentralized, meaning that they are not controlled by any central authority. This makes them more secure and less prone to manipulation or censorship.
- Liquidity – ERC20 tokens can be easily traded on decentralized exchanges or other trading platforms. This can make it easier for investors and users to buy and sell their tokens as needed.
- Ecosystem – There is an entire DeFi and CeFi ecosystem built around the ERC20 standard. Things like Uniswap, Metamask, Etherscan all have built in functionality to support newly minted ERC20 tokens.
Solidity developers can leverage this standard to create their own tokens and integrate them with other decentralized applications, building on the lego bricks of DeFi.
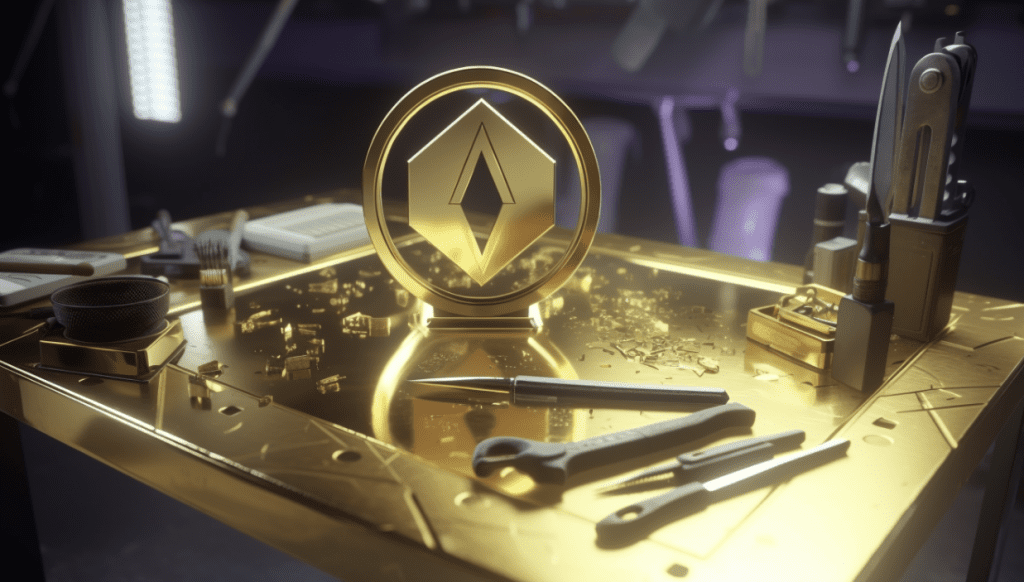
Simple ERC20 Token Contract
This code is available in the Solidity Snippets Github Repository: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/ERC20.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor() ERC20("MyToken", "MYTKN") {
_mint(msg.sender, 1000000 ether);
}
}
The OpenZeppelin Library
Note that in the above code we are importing the OpenZeppelin library which provides all the functionality we need for our token.
It’s worth having a read through the library contract alongside the function descriptions below to get a good idea of how the logic works.
https://github.com/OpenZeppelin/openzeppelin-contracts/blob/master/contracts/token/ERC20/ERC20.sol
There is a useful preset in token/ERC20/presets/ERC20PresetMinterPauser.sol which provides additional functionality in the form of making the token mintable, burnable and pausable based on AccessControlEnumerable.sol
There are also a whole host of extensions for the ERC20 token
- ERC20Mintable – allows for the creation of new tokens by specific accounts with MinterRole permission.
- ERC20Burnable – enables token holders to destroy their tokens or those they have an allowance for through a burn function.
- ERC20Pausable – allows for pausing and unpausing token transfers and allowances. Useful for stopping trades during a crowdsale or in the event of an emergency.
- ERC20Capped – adds a cap to the total supply of tokens. Only accounts with MinterRole permission can create new tokens, subject to the cap.
ERC20 Token Functions
Here is a list of all the standard ERC20 functions and events and what they do. External functions can be called from the frontend or anywhere else whereas internal functions are available only within the contract itself.
External ERC20 Functions
totalSupply()
Returns the total number of tokens in existence within the smart contract.balanceOf(account)
Returns the token balance of a specific account.- transfer(recipient, amount): Transfers a certain number of tokens from the sender’s account to the recipient’s account.
allowance(owner, spender)
Returns the amount of tokens that the spender is allowed to spend on behalf of the owner account.approve(spender, amount)
Sets the allowance of the spender account to a certain amount of tokens.transferFrom(sender, recipient, amount)
Transfers a certain number of tokens from the sender account to the recipient account, on the condition that the spender account has been authorized to spend the specified number of tokens.increaseAllowance(spender, addedValue)
This function increases the amount of tokens that a spender is allowed to transfer on behalf of an owner.decreaseAllowance(spender, subtractedValue)
This function decreases the amount of tokens that a spender is allowed to transfer on behalf of an owner.
Internal ERC20 Functions
_transfer(sender, recipient, amount)
This function is used internally to transfer tokens from one address to another. It is called by the transfer and transferFrom functions._mint(account, amount)
This function is used internally to mint new tokens and add them to an account’s balance. It is called by the mint function in ERC20Mintable._burn(account, amount)
This function is used internally to burn (remove) tokens from an account’s balance. It is called by the burn function in ERC20Burnable._approve(owner, spender, amount)
This function is used internally to set the allowance for a spender on behalf of an owner. It is called by the approve function._burnFrom(account, amount)
This function is used internally to burn tokens from an account’s balance on behalf of a spender. It is called by the burnFrom function in ERC20Burnable.
ERC20 Events
Transfer(from, to, value)
This event is emitted whenever tokens are transferred from one address to another. It indicates the amount of tokens transferred (value), the address of the sender (from), and the address of the recipient (to).Approval(owner, spender, value)
This event is emitted whenever the allowance of a spender is updated by the owner of the tokens. It indicates the amount of tokens approved (value), the address of the token owner (owner), and the address of the approved spender (spender).
Conclusion
ERC20 tokens have become the industry standard for token implementation on the Ethereum blockchain. They provide a common set of rules for creating and managing tokens which enables developers to easily create, deploy and interact with the DeFi ecosystem.
As a Solidity developer building on Ethereum, understanding and utilizing the ERC20 standard is crucial because almost all projects will utilize tokens in one way or another. By studying this standard, developers can ensure that they have a thorough understanding of one of the most important tools in a blockchain devs toolbox.
The popularity of the ERC20 standard has led to the creation of many tools, frameworks and libraries that simplify the process of developing ERC20 tokens. The OpenZeppelin libraries make it easy to create and deploy your token with ease.
The ERC20 standard has proven to be an essential building block for the Ethereum ecosystem, enabling the creation of new decentralized economies and financial systems.
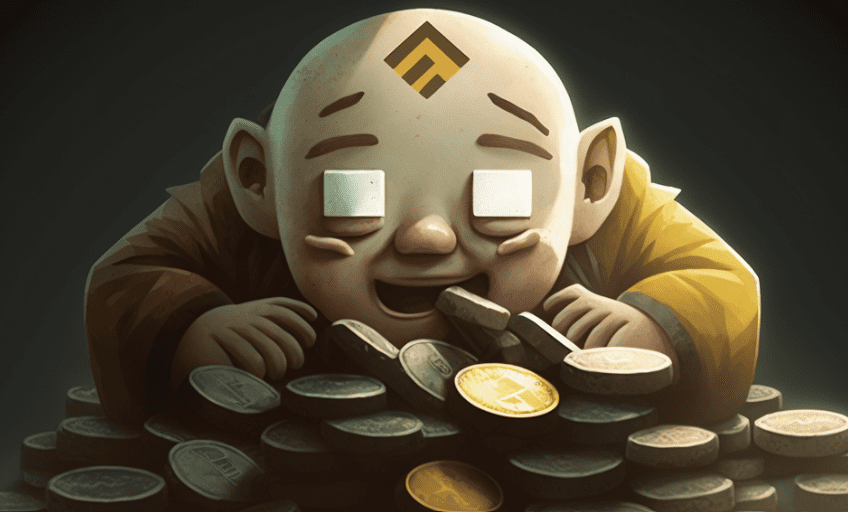