A Solidity Event is a way for smart contracts to communicate with the outside world by providing a mechanism for emitting messages that can be observed by external applications. It’s like a signalling message that is broadcast when a certain condition is met within the smart contract.
Emitting Events In Solidity
Here is a simple example of how to use events:
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
contract Events {
event LogThis(string msg);
function test() external {
emit LogThis('Hello World');
}
}
Full code is available in the Solidity Snippets Github Repo: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Events.sol
Note that the event itself is declared at the very top level of the contract alongside the state variables. It is then emitted with some data within a function.
Monitoring Solidity Events
When an event is emitted, it creates a log entry in the blockchain. These logs can be filtered and searched by external applications, allowing them to monitor the behaviour of the smart contract. Something like Dune Analytics is a good example of how you can set up a 3rd party RPCnode/database client to monitor events on a verified contract.
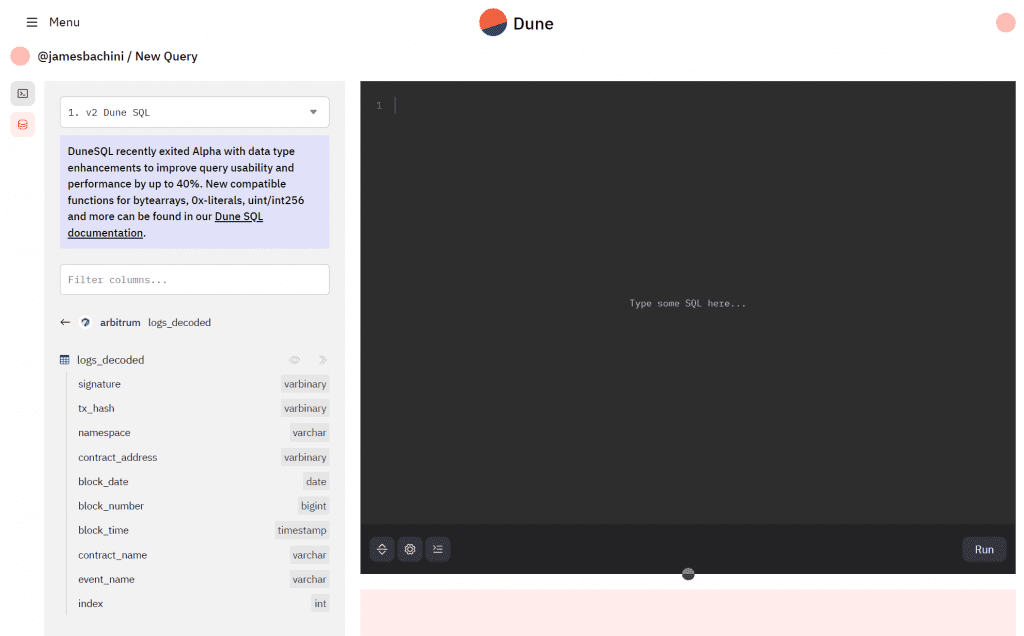
Events are logged in a separate data storage that can be read easily by nodes on the network. We can listen to the events being emitted by subscribing to it from our frontend. In this example we are using ethers.js to subscribe to the event from the above contract:
filter = {
address: 0xContractAddressHere,
topics: [
LogThis(string)
]
}
provider.on(filter, (e) => {
console.log(e);
})
Here we use the “provider.on()” method to do something everytime the LogThis event is fired.
When we subscribe to events we create a callback which helps to avoid the need for multiple reloads to check transaction state which can be inefficient.
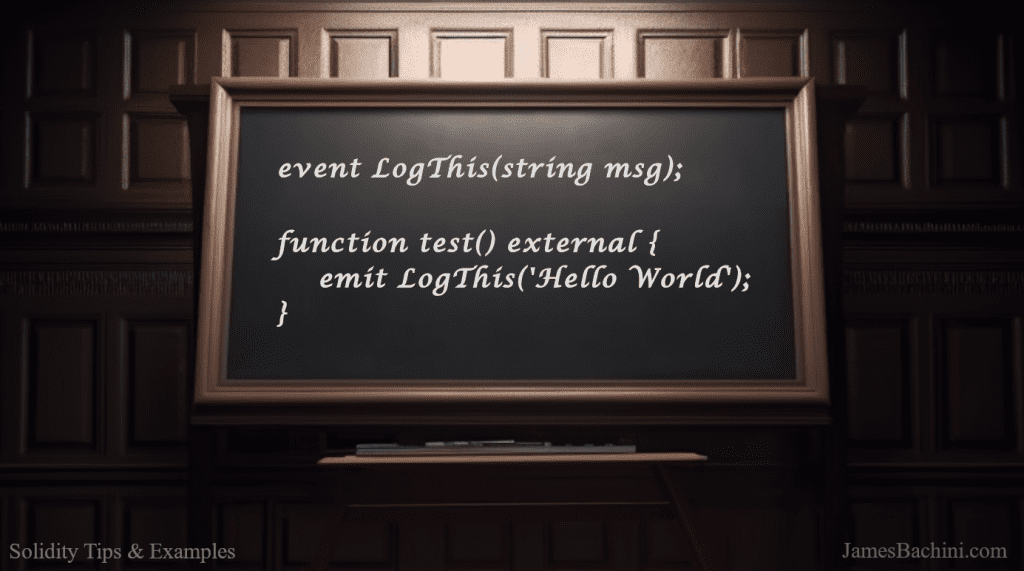
Good Practices For Solidity Events
I’ll be honest here, when I’m developing code, adding events comes as an afterthought and something that is added immediately prior to starting unit tests as I tidy up the mess of a smart contract I’ve just created.
I think it’s good to have a stage in your development process where you look at code quality and during this stage it’s worth going through the events your contract has and what might be needed in the future.
I like to add an event whenever a state changing function is called and generally log the input data to that function. For example if someone stakes funds in a vault that should fire an event with the quantity of funds they have staked.
The name of the event will generally follow the name of the function but with a capital starting letter i.e. event StakeFunds(uint _amount);
This allows for the frontend devs and other 3rd parties to easily access the amount of funds going into a pool and generate historical data for charts etc.
I hope that this introduction to emitting events in Solidity has been of interest and one day helps avoid the feeling of despair when you realise you can’t get the data you need because there are no events logged in the contract you deployed last week.