To get the contract balance in solidity there are a few different methods depending on if you want the Ether balance or an ERC20 token balance.
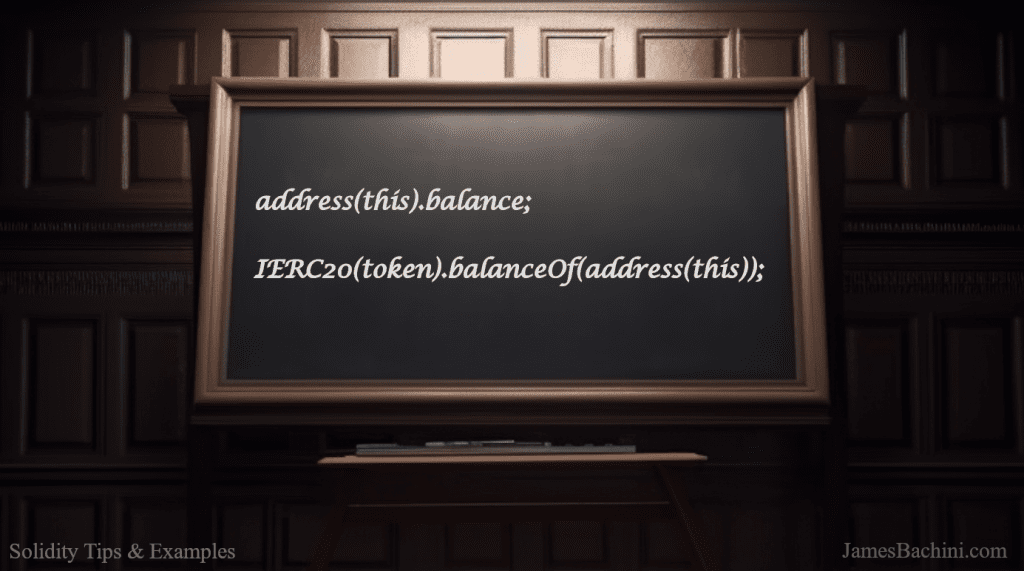
Contract Balance Eth
You can use the address type’s balance property to get the balance of any address, including the contract’s own address. The this value is used to define the contract itself.
To get the Ether balance of your contract, you can use the following code:
address(this).balance;
The balance of the address is returned in Wei, which is the smallest unit of ether in Ethereum, 1 Ether = 1**18 wei (i.e. ether has 18 decimals).
Contract Balance ERC20
To get the ERC20 balance or token balance we need to import an ERC interface to use the balanceOf query. We then need to define a token contract address and user address or contract address to check.
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
interface IERC20 {
function balanceOf(address account) external view returns (uint);
}
contract BalanceExample {
address private wethTokenAddress = 0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2;
uint public wethBalance = IERC20(wethTokenAddress).balanceOf(address(this));
}
This is a simple Solidity smart contract using a simplified IERC20
interface, which is an interface for the ERC-20 token standard on Ethereum. The purpose of this contract is to get the balance of a specific ERC-20 token for the contract’s address.
Note that it is also possible to import the OpenZeppelin IERC20 full interface including all ERC20 token function using this code:
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
Solidity Contract Balance Example
There is a full example contract which demonstrates these contract balance functions available in the Github Solidity Snippets repo:
https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/ContractBalance.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
contract BalanceExample {
uint public ethBalance = address(this).balance;
address private wethTokenAddress = 0xC02aaA39b223FE8D0A0e5C4F27eAD9083C756Cc2;
uint public wethBalance = IERC20(wethTokenAddress).balanceOf(address(this));
}