As an experiment I converted this Vyper token contract to a Javascript/Typescript syntax to see if we could make it easier for web developers to get up to speed with smart contract development.
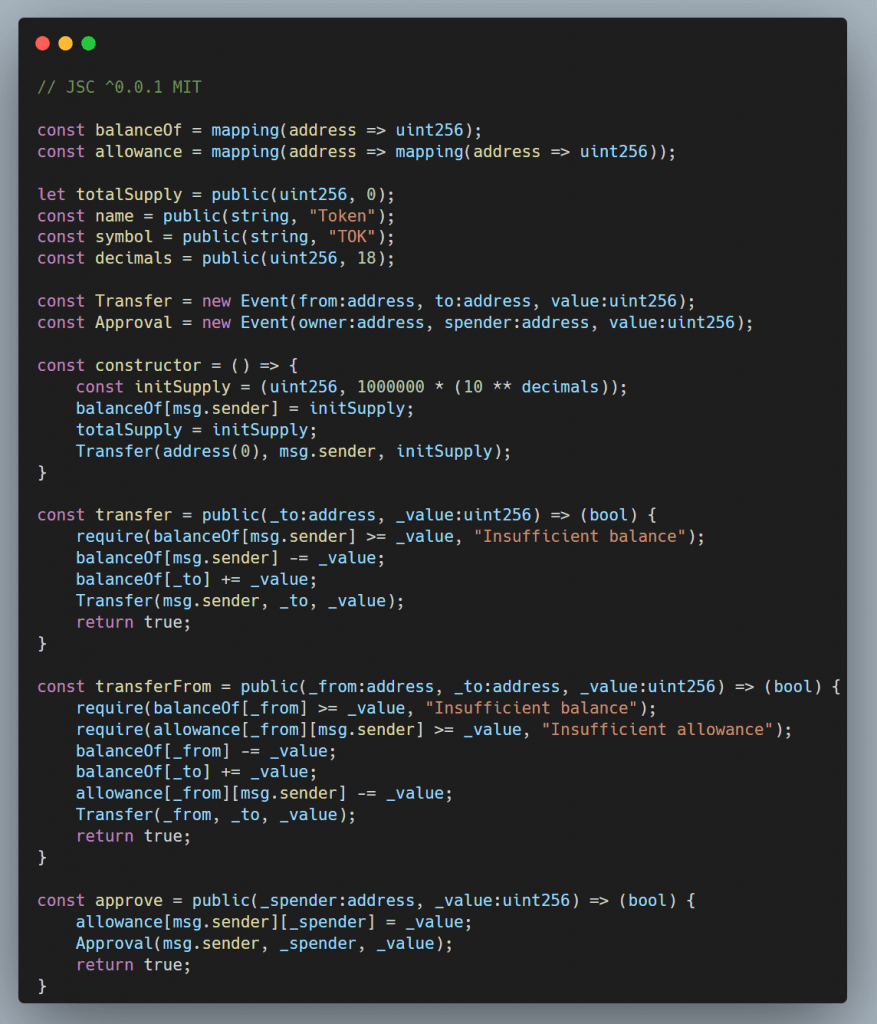
Solidity is the most popular smart contract language and it is already based losely on Javascript but there are plenty of syntax differences and quirks that have crept in over it’s decade long existence.
In theory you could have a new language like the above which can either compile directly to byte code or be converted easily to Solidity and then bytecode using the latest compiler prior to deployment.
If web devs don’t need to learn a new language then it would remove some of the roadblocks and make adoption of blockchain technology easier. It could also be used as a stepping stone language for beginner/younger developers.
“Any application that can be written in JavaScript, will eventually be written in JavaScript.”
Atwoods Law
To build this out it would need a compiler and some significant work on error handling and testing. It would likely also need integration support from at least Etherscan and Remix which in turn would probably require support from the Ethereum Foundation.
ChatGPT can convert this format directly to Solidity although that’s not robust enough for a production level compiler. If you copy and paste the below code in to ChatGPT with the prompt “convert this contract to Solidity” it compiles fine and can be deployed via remix.
Perhaps an idea for the future…
// JSC ^0.0.1 MIT
const balanceOf = mapping(address => uint256);
const allowance = mapping(address => mapping(address => uint256));
let totalSupply = public(uint256, 0);
const name = public(string, "Token");
const symbol = public(string, "TOK");
const decimals = public(uint256, 18);
const Transfer = new Event(from:address, to:address, value:uint256);
const Approval = new Event(owner:address, spender:address, value:uint256);
const constructor = () => {
const initSupply = (uint256, 1000000 * (10 ** decimals));
balanceOf[msg.sender] = initSupply;
totalSupply = initSupply;
Transfer(address(0), msg.sender, initSupply);
}
const transfer = public(_to:address, _value:uint256) => (bool) {
require(balanceOf[msg.sender] >= _value, "Insufficient balance");
balanceOf[msg.sender] -= _value;
balanceOf[_to] += _value;
Transfer(msg.sender, _to, _value);
return true;
}
const transferFrom = public(_from:address, _to:address, _value:uint256) => (bool) {
require(balanceOf[_from] >= _value, "Insufficient balance");
require(allowance[_from][msg.sender] >= _value, "Insufficient allowance");
balanceOf[_from] -= _value;
balanceOf[_to] += _value;
allowance[_from][msg.sender] -= _value;
Transfer(_from, _to, _value);
return true;
}
const approve = public(_spender:address, _value:uint256) => (bool) {
allowance[msg.sender][_spender] = _value;
Approval(msg.sender, _spender, _value);
return true;
}