This article explores how mapping in Solidity is used to for storing key->value pairs, where the keys are of a specific data type and the values can be of any data type.
Mappings are declared using the mapping keyword, followed by the key data type in parentheses and the value data type after the parentheses.
mapping(address => uint256) public balances;
In this example, we have declared a mapping called balances that maps address values to uint256 values. This mapping is declared as public, which means that it can be read by any other contract or externally through a automatically generated getter function.
To add a key-value pair to a mapping, we use the key as the index of the mapping and assign a value to it.
balances[msg.sender] = 420;
Here we are setting the balance of msg.sender to 420. The msg.sender variable represents the address of the account that called the smart contract function.
Mappings are particularly useful for keeping track of data associated with specific addresses or other data types. For example, we can use a mapping to keep track of the balance of each address in a cryptocurrency smart contract, or to keep track of the ownership of non-fungible tokens (NFTs).
mapping(id => address) public owners;
It’s important to note that mappings are unordered, which means that there is no guaranteed order in which the key value pairs are stored or retrieved.
Mappings cannot be iterated over, which means that we cannot access all the key-value pairs in a mapping at once. However, we can use additional data structures like arrays or loops to perform operations on all the key-value pairs in a mapping.
Mapping In Solidity Code Example
The code for this example is also available on Github: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Mapping.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
// Mapping Addresses To Balances
contract AddressMap {
mapping(address => uint) public balances;
function addFunds() public {
balances[msg.sender] = 1000;
}
function checkFunds(address _addr) public view returns(uint) {
return balances[_addr];
}
}
I hope this short example of how to use mappings in Solidity has been of interest and provides useful examples of how to use mapping in your code.
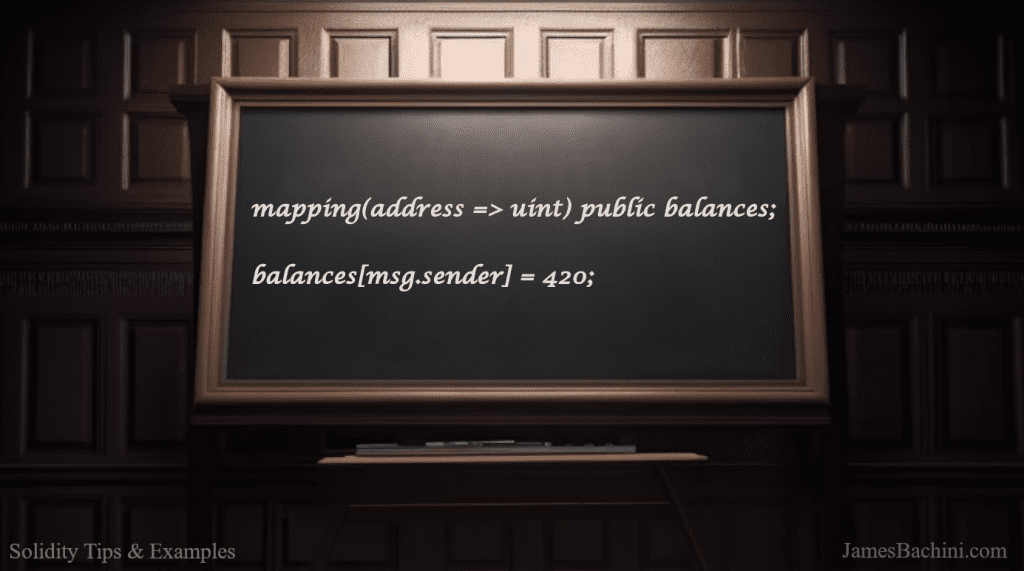