Payable transfers are one of a number of ways to send ether from a Solidity smart contract to an external address.
payable(jamesAddress).transfer(1 Ether);
In this next example we create a fallback function so that any user can send this contract ether and it will get split between the different addresses.
Full code at: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/PaymentDivider.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract Distributor {
address developer = 0xAb8483F64d9C6d1EcF9b849Ae677dD3315835cb2;
address lawfirm = 0x4B20993Bc481177ec7E8f571ceCaE8A9e22C02db;
address bizdev = 0x78731D3Ca6b7E34aC0F824c42a7cC18A495cabaB;
fallback() external payable {
uint developerAmount = msg.value * 90 / 100;
uint lawfirmAmount = msg.value * 5 / 100;
uint bizdevAmount = msg.value - developerAmount - lawfirmAmount;
payable(developer).transfer(developerAmount);
payable(lawfirm).transfer(lawfirmAmount);
payable(bizdev).transfer(bizdevAmount);
}
}
Payable fails if the transfer does not go through and reverts the transaction. If you want to return a boolean instead use .send(uint)
bool sent = payable(developer).send(developerAmount);
The current recommended way to send ether according to the docs which potentially opens up more flexibility to send and receive data is to use .call()
(bool sent, bytes memory data) = payable(developer).call{value: developerAmount}("");
A contract receiving ether must have a fallback or receiver function to allow for it to receive ether. Note that it is still possible to force Ether on a contract using the selfdestruct vulnerability hack.
receive() external payable {}
fallback() external payable {}
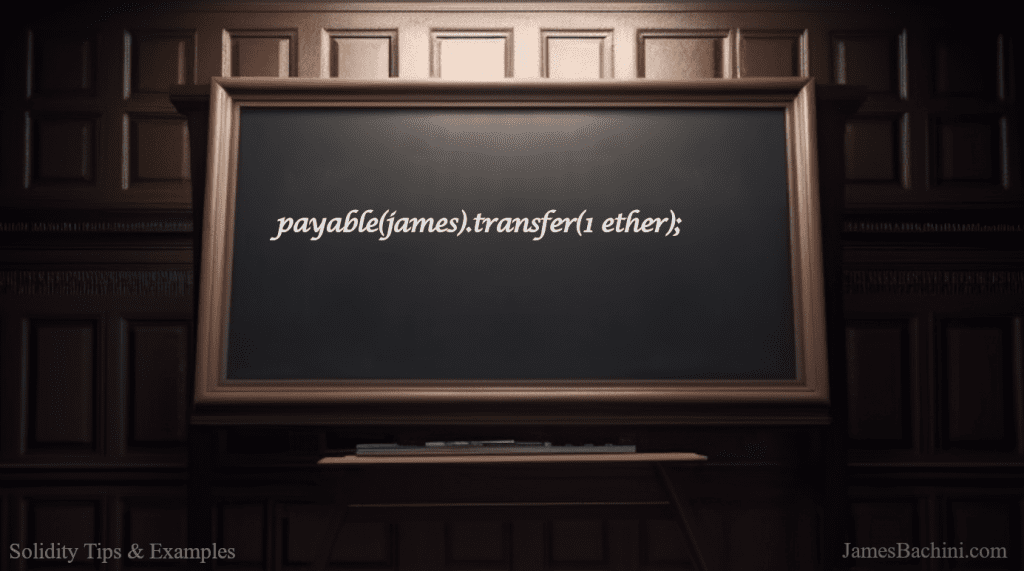