This single file server health check script works on Windows, Linux and possibly others. It is written in PHP to show CPU usage, RAM usage, Incoming Connections and Hard disk usage.
Update September 2020 Server Check PHP was getting a lot of downloads so I’ve updated the code and open sourced it on Github.
If you want to know the functions to get RAM & CPU usage from within PHP jump to the code section here.
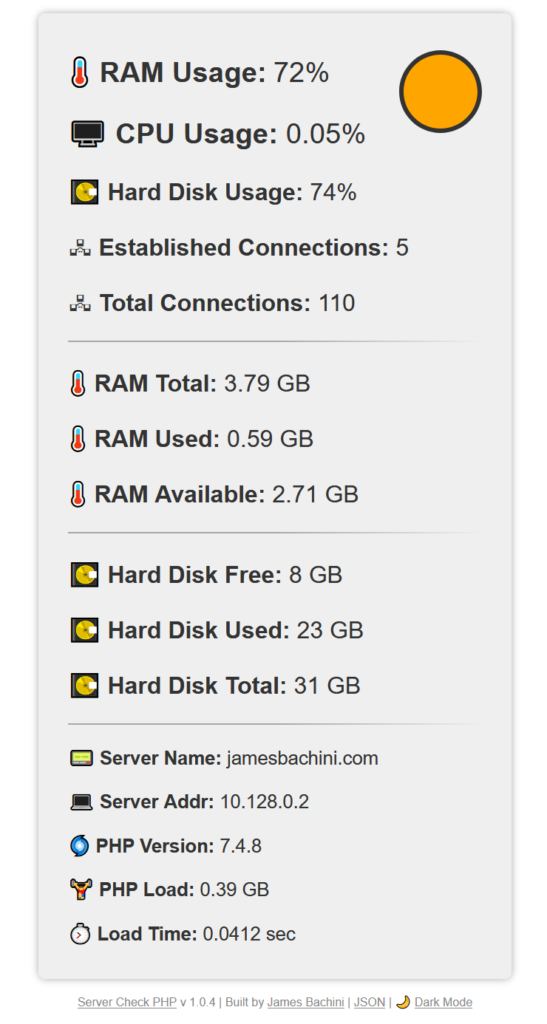
Server Check demo here: https://jamesbachini.com/misc/servercheck.php
JSON output: https://jamesbachini.com/misc/servercheck.php?json=1
Single file download here: https://jamesbachini.com/misc/servercheck.zip
Github Repo here: https://github.com/jamesbachini/Server-Check-PHP
Download this script to display RAM & CPU usage for your server.
Building A Server Health Check PHP Script
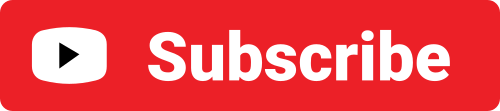
PHP Ram Usage & PHP CPU Usage Code
The functionality is different for Windows and Linux so the first thing we need to do is determine the operating system.
$operating_system = PHP_OS_FAMILY;
if ($operating_system === 'Windows') {
Then I used the Windows management interface to determine the RAM and CPU usage. Netstat command works on both Windows and Linux although the formatting is different.
// Win CPU
$wmi = new COM('WinMgmts:\\\\.');
$cpus = $wmi->InstancesOf('Win32_Processor');
$cpuload = 0;
$cpu_count = 0;
foreach ($cpus as $key => $cpu) {
$cpuload += $cpu->LoadPercentage;
$cpu_count++;
}
// WIN MEM
$res = $wmi->ExecQuery('SELECT FreePhysicalMemory,FreeVirtualMemory,TotalSwapSpaceSize,TotalVirtualMemorySize,TotalVisibleMemorySize FROM Win32_OperatingSystem');
$mem = $res->ItemIndex(0);
$memtotal = round($mem->TotalVisibleMemorySize / 1000000,2);
$memavailable = round($mem->FreePhysicalMemory / 1000000,2);
$memused = round($memtotal-$memavailable,2);
// WIN CONNECTIONS
$connections = shell_exec('netstat -nt | findstr :80 | findstr ESTABLISHED | find /C /V ""');
$totalconnections = shell_exec('netstat -nt | findstr :80 | find /C /V ""');
Then for Linux we have sys_getloadavg() available for CPU usage within PHP. Then for RAM I used the “free” shell command.
// Linux CPU
$load = sys_getloadavg();
$cpuload = $load[0];
// Linux MEM
$free = shell_exec('free');
$free = (string)trim($free);
$free_arr = explode("\n", $free);
$mem = explode(" ", $free_arr[1]);
$mem = array_filter($mem, function($value) { return ($value !== null && $value !== false && $value !== ''); }); // removes nulls from array
$mem = array_merge($mem); // puts arrays back to [0],[1],[2] after
$memtotal = round($mem[1] / 1000000,2);
$memused = round($mem[2] / 1000000,2);
$memfree = round($mem[3] / 1000000,2);
$memshared = round($mem[4] / 1000000,2);
$memcached = round($mem[5] / 1000000,2);
$memavailable = round($mem[6] / 1000000,2);
// Linux Connections
$connections = `netstat -ntu | grep :80 | grep ESTABLISHED | grep -v LISTEN | awk '{print $5}' | cut -d: -f1 | sort | uniq -c | sort -rn | grep -v 127.0.0.1 | wc -l`;
$totalconnections = `netstat -ntu | grep :80 | grep -v LISTEN | awk '{print $5}' | cut -d: -f1 | sort | uniq -c | sort -rn | grep -v 127.0.0.1 | wc -l`;
For the hard disk usage I found PHP native functions that worked across operating systems:-
$diskfree = round(disk_free_space(".") / 1000000000);
$disktotal = round(disk_total_space(".") / 1000000000);
$diskused = round($disktotal - $diskfree);
$diskusage = round($diskused/$disktotal*100);
Full source code is available here: https://github.com/jamesbachini/Server-Check-PHP
If you are interested in online business, performance marketing and web development then check out my Twitter and Youtube Channel.
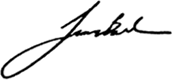
————————- original post below —————————–
I needed a script to do a basic server health check and built the following which can be viewed here:
http://jamesbachini.com/misc/ram.php
and downloaded here:
http://jamesbachini.com/misc/ram.zip
It measures RAM, CPU, Hard disk usage, Established and total connections, a few other bits and pieces. It has a traffic light system which is setup for my needs but you can fiddle with as necessary if you want to change the parameters.
Here’s a screenshot:
To install just unzip the package and upload the ram.php file to your server. Then visit http://myserver.com/myfolder/ram.php or wherever you put it in your normal web/mobile browser.
And here’s the full PHP code, just in case you are interested:
$server_check_version = '1.0.4';
$start_time = microtime(TRUE);
$operating_system = PHP_OS_FAMILY;
if ($operating_system === 'Windows') {
// Win CPU
$wmi = new COM('WinMgmts:\\\\.');
$cpus = $wmi->InstancesOf('Win32_Processor');
$cpuload = 0;
$cpu_count = 0;
foreach ($cpus as $key => $cpu) {
$cpuload += $cpu->LoadPercentage;
$cpu_count++;
}
// WIN MEM
$res = $wmi->ExecQuery('SELECT FreePhysicalMemory,FreeVirtualMemory,TotalSwapSpaceSize,TotalVirtualMemorySize,TotalVisibleMemorySize FROM Win32_OperatingSystem');
$mem = $res->ItemIndex(0);
$memtotal = round($mem->TotalVisibleMemorySize / 1000000,2);
$memavailable = round($mem->FreePhysicalMemory / 1000000,2);
$memused = round($memtotal-$memavailable,2);
// WIN CONNECTIONS
$connections = shell_exec('netstat -nt | findstr :80 | findstr ESTABLISHED | find /C /V ""');
$totalconnections = shell_exec('netstat -nt | findstr :80 | find /C /V ""');
} else {
// Linux CPU
$load = sys_getloadavg();
$cpuload = $load[0];
// Linux MEM
$free = shell_exec('free');
$free = (string)trim($free);
$free_arr = explode("\n", $free);
$mem = explode(" ", $free_arr[1]);
$mem = array_filter($mem, function($value) { return ($value !== null && $value !== false && $value !== ''); }); // removes nulls from array
$mem = array_merge($mem); // puts arrays back to [0],[1],[2] after
$memtotal = round($mem[1] / 1000000,2);
$memused = round($mem[2] / 1000000,2);
$memfree = round($mem[3] / 1000000,2);
$memshared = round($mem[4] / 1000000,2);
$memcached = round($mem[5] / 1000000,2);
$memavailable = round($mem[6] / 1000000,2);
// Linux Connections
$connections = `netstat -ntu | grep :80 | grep ESTABLISHED | grep -v LISTEN | awk '{print $5}' | cut -d: -f1 | sort | uniq -c | sort -rn | grep -v 127.0.0.1 | wc -l`;
$totalconnections = `netstat -ntu | grep :80 | grep -v LISTEN | awk '{print $5}' | cut -d: -f1 | sort | uniq -c | sort -rn | grep -v 127.0.0.1 | wc -l`;
}
$memusage = round(($memavailable/$memtotal)*100);
$phpload = round(memory_get_usage() / 1000000,2);
$diskfree = round(disk_free_space(".") / 1000000000);
$disktotal = round(disk_total_space(".") / 1000000000);
$diskused = round($disktotal - $diskfree);
$diskusage = round($diskused/$disktotal*100);
if ($memusage > 85 || $cpuload > 85 || $diskusage > 85) {
$trafficlight = 'red';
} elseif ($memusage > 50 || $cpuload > 50 || $diskusage > 50) {
$trafficlight = 'orange';
} else {
$trafficlight = '#2F2';
}
$end_time = microtime(TRUE);
$time_taken = $end_time - $start_time;
$total_time = round($time_taken,4);
// use servercheck.php?json=1
if (isset($_GET['json'])) {
echo '{"ram":'.$memusage.',"cpu":'.$cpuload.',"disk":'.$diskusage.',"connections":'.$totalconnections.'}';
exit;
}