What happens when you ask a blockchain developer to sell you a pen?
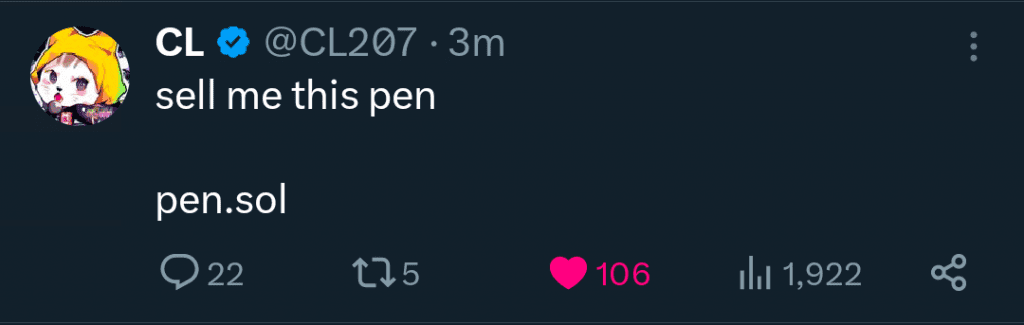
Etch your words permanently on the Ethereum blockchain where they will outlive you & create an everlasting record of your thoughts, contemplations & predictions.
This is your chance to leave an eternal mark and express your presence in the digital age while being rewarded with a “Write to Earn” token.
Deployed to Ethereum: 0x7eae7422f633429EE72BA991Ac293197B80D5976
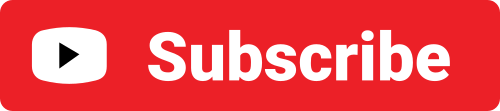
To create a Pen smart contract I wanted to pop an event and store a string of text for each user. I also wanted to add some degen tokenomics to incentivize users to participate.
This is what I came up with. The full code is also available on Github here: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Pen.sol
I’ll go through some of the interesting bits here:
uint maxSupply = 1_000_000 * 10 ** decimals();
uint reward = 1_000 * 10 ** decimals();
mapping(address => string) public paper;
mapping(address => bool) public rewarded;
event LogThis(string msg);
We are initially setting a max supply of 1m tokens and distributing 1000 to each user. The paper variable maps a string to each users address, this is overwritten on each use. The rewarded variable maps a true/false boolean to the user if they have already received a token reward.
The LogThis event creates a permanent record of every update which can be tracked by 3rd party services and block explorers.
function write(string memory _msg) public {
paper[msg.sender] = _msg;
emit LogThis(_msg);
distributeRewards();
}
The write() function is public and enables anyone to store a text message on the Ethereum blockchain. It pops the event which logs the message and also calls an internal function distributeRewards();
function distributeRewards() internal {
if (totalSupply() + reward < maxSupply && rewarded[msg.sender] == false) {
rewarded[msg.sender] = true;
_mint(msg.sender, reward);
}
}
This function checks if the reward will push the supply over the maxSupply and makes sure the user hasn’t already received a reward before calling _mint(user, amount) which is an ERC20 internal function provided by the OpenZeppelin library to mint new tokens.
All in all it’s a pretty simple contract with under 30 lines of code that has a better use case than many of the billion dollar blockchain projects listed on Coinmarketcap.
That being said don’t expect to get rich with the PEN token as there isn’t a Uniswap pool and it holds no value at time of writing. This project is a good demonstration of the immutable data storage potential inherent in blockchain systems and how powerful it can be for devs to deploy code to a decentralized peer to peer network.
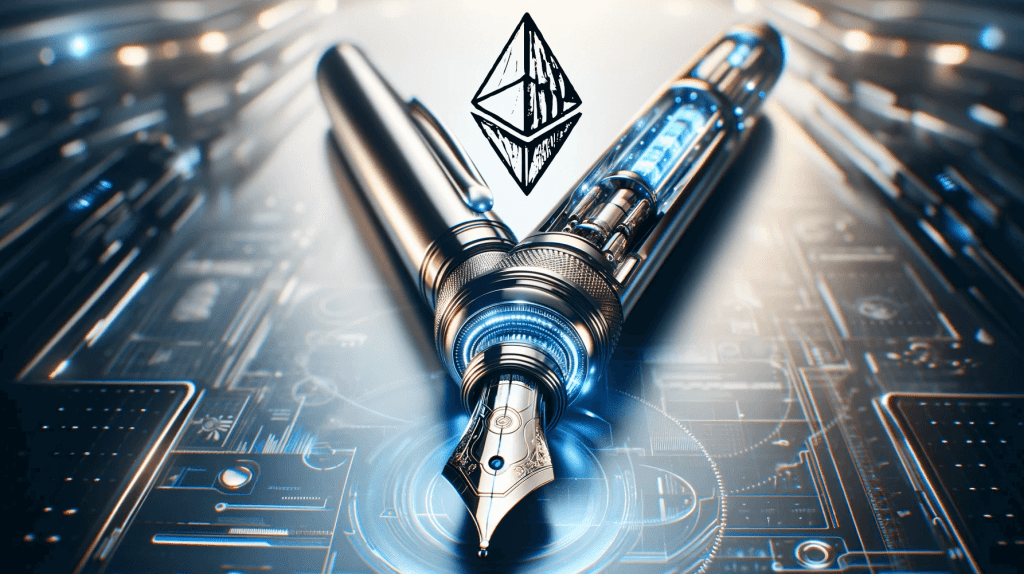
– The Pen Token