A Solidity interface is code that provides a set of function declarations without any implementation details. Interfaces are used to interact with 3rd party contracts or external systems by defining a common set of functions that both parties agree to implement.
Interfaces are used to define a contract’s external-facing functions, which is the only part of the contract that can be accessed when building on the lego bricks of DeFi.
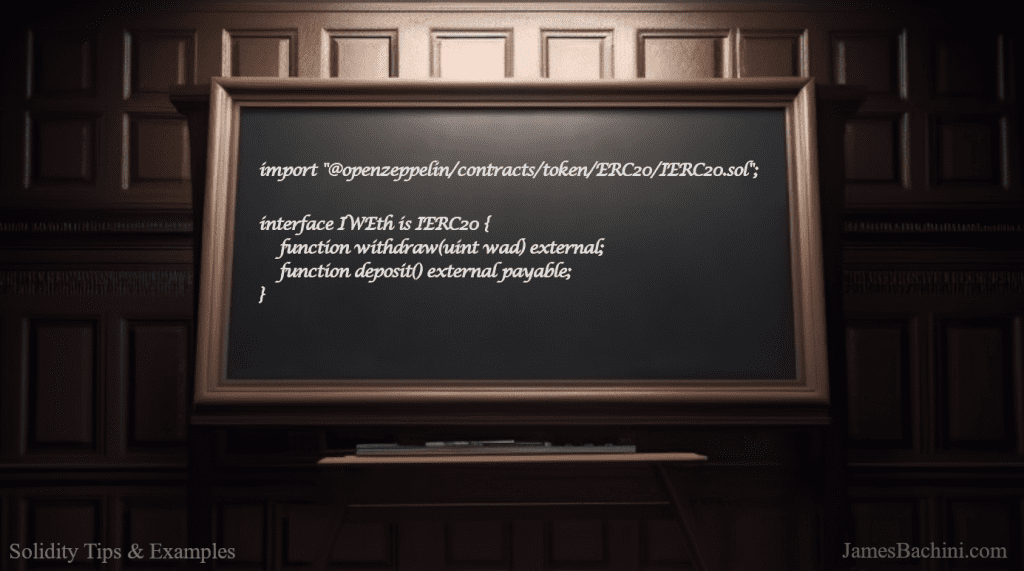
Here is an example of an interface for an ERC20 token which defines the token functions that we have available.
interface IERC20 {
function totalSupply() external view returns (uint);
function balanceOf(address account) external view returns (uint);
function transfer(address recipient, uint amount) external returns (bool);
function allowance(address owner, address spender) external view returns (uint);
function approve(address spender, uint amount) external returns (bool);
function transferFrom(address sender, address recipient, uint amount) external returns (bool);
event Transfer(address indexed from, address indexed to, uint value);
event Approval(address indexed owner, address indexed spender, uint value);
}
This interface is defined using the interface keyword. Notice that each function is written very similarly to how you would write a function header in Solidity i.e.
function balanceOf(address account) external view returns (uint) {
return _balances[account];
}
// can be converted into an interface below:
function balanceOf(address account) external view returns (uint);
To use an interface, a contract can inherit from it and implement the required functions. This way, the contract can be used as if it implements the interface. In the below example we import an openzeppelin interface library instead of defining it ourselves.
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
contract Test {
address internal tokenAddress = 0x0;
uint public bal = IERC20(tokenAddress).balanceOf(address(this));
}
An interface can be implemented by any contract that provides the same set of functions with the same input and output types. This allows for easy standardized communication between contracts and makes it possible to integrate with other smart contracts and external systems in a modular way.
By using interfaces developers can build on existing DeFi protocols and create new products by connecting the lego bricks of DeFi