A solidity library is a reusable contract that contains functions that can be called by other contracts. When you import a Solidity library into your contract, you can use the functions provided by the library.
- How To Import Solidity Libraries
- Popular Solidity Libraries
- Create Your Own Solidity Library
- Conclusion
How To Import Solidity Libraries
In your smart contract, you can import the library by using the import keyword followed by the file name of the library. Note that you either need to use a locally hosted library file or a external library like OpenZeppelin.
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor() ERC20("MyToken", "MYTKN") {
_mint(msg.sender, 1000000 ether);
}
}
The code above imports an ERC20 library and uses this to create MyToken.
If you have errors when compiling with library not found, try installing the OpenZeppelin libraries from the command line
npm install @openzeppelin/contracts
Popular Solidity Libraries
OpenZeppelin – My favourite collection of Solidity templates and utilities.
https://github.com/OpenZeppelin/openzeppelin-contracts/tree/master/contracts
Third Web – A collection of libraries for tokens, staking, drops etc.
https://github.com/thirdweb-dev/contracts/tree/main/contracts
Create Your Own Solidity Library
Here is an example of how you can create your own Solidity library. Let’s first create the library in a file called TestLibrary.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
library TestLibrary {
function subtract(uint a, uint b) internal pure returns (uint) {
return a - b;
}
}
Then we can create a TestContract.sol file and import the library. Note that there is an example of this code importing into the same file in the Solidity Snippets Github Repo
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "./TestLibrary.sol";
contract MyContract {
function func(uint a, uint b) public pure returns (uint) {
return TestLibrary.subtract(a, b);
}
}
In the above example we are importing the library we just created and using the TestLibrary.subtract(a, b)
function from the new library. Note that the internal
visibility modifier is used in the library function to ensure that it can only be called by contracts that inherit from the library. This is because library functions cannot be called by external accounts or contracts.
Conclusion
Solidity libraries provide an effective way to write modular and expandable code in smart contract development. By creating reusable libraries for specific functions, developers can avoid duplication of code and reduce the risk of errors, resulting in more efficient and secure code.
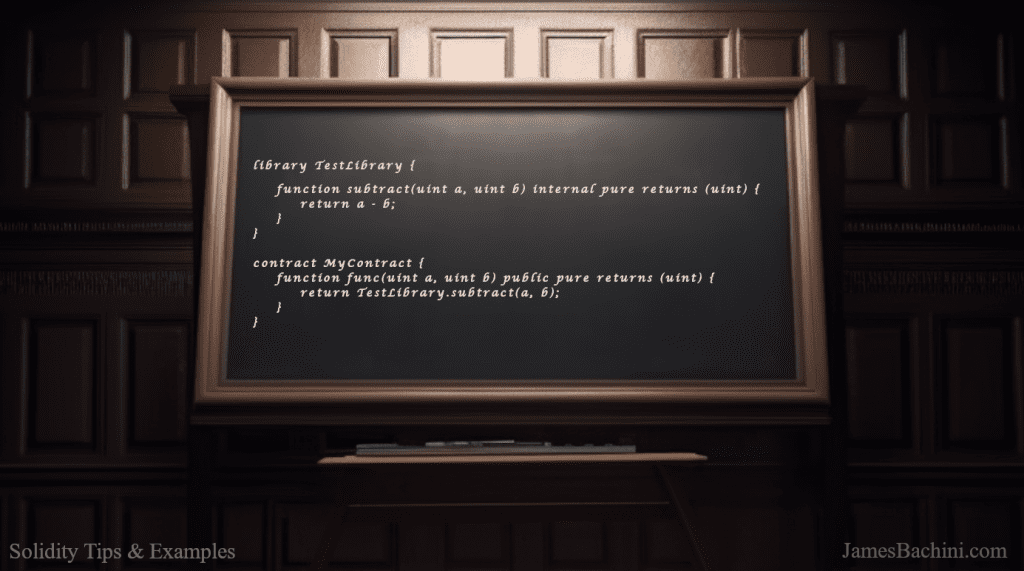
The use of libraries can also promote collaboration between developers, as multiple contracts can rely on a single library to perform a specific task. By embracing the use of Solidity libraries, developers can build more robust and scalable decentralized applications that meet the needs of their users.