A string in Solidity is a data type used to store text. Working with strings in Solidity can be challenging due to the limitations and efficiency required by the Ethereum virtual machine. This article aims to provide a comprehensive guide on how to use strings in Solidity, covering their properties, limitations, and best practices for manipulating and storing them in smart contracts.
Solidity Strings
Here is an example of how to define a string in Solidity
string public myVariable = 'Hello World';
A string is a sequence of characters represented in UTF-8 encoding. It can contain any Unicode character and has no fixed size, meaning it can grow dynamically as needed.
In terms of usage, Solidity strings are similar to strings in other programming languages, such as JavaScript or Python. You can access individual characters using array notation (e.g. myString[0]
).
Escape Characters
Escape characters are used in Solidity strings to represent special characters or sequences of characters that would otherwise be difficult or impossible to include directly in the string. Here is how each of the escape characters you listed works:
\<newline> This escape sequence is used to insert an actual newline character into a string. It is commonly used to break a long string into multiple lines for readability.
\’ This escape sequence is used to insert a single quote character into a string. Similar to the backslash, the single quote is a special character and needs to be escaped to be used as a regular character in a string.
\” This escape sequence is used to insert a double quote character into a string. Double quotes are also special characters that need to be escaped in order to be used as a regular character.
\n This escape sequence is used to insert a newline character into a string. It is similar to the <newline> sequence but represents a newline character as a single character rather than as an escape sequence.
\r This escape sequence is used to insert a carriage return character into a string. It is another way to represent a newline character, but is less commonly used in Solidity.
\t This escape sequence is used to insert a tab character into a string. It is commonly used to align text in a table or to add whitespace between characters.
\xNN This escape sequence is used to insert a byte value represented in hexadecimal notation into a string. The value is specified by the two hexadecimal digits after the ‘x’. For example, \x41 represents the ASCII character ‘A’.
\uNNNN This escape sequence is used to insert a Unicode character represented in UTF-8 notation into a string. The value is specified by the four hexadecimal digits after the ‘u’.
For example, \u00A9 represents the copyright symbol ©.
Working With Strings
From Solidity compiler version 0.8.12 we can use the following to add one string on to the end of another.
string s1 = 'Hello ';
string s2 = 'World';
string s3 = string.concat(s1, s2);
Prior to that we would use something like this which is still widely used
string s3 = string(abi.encodePacked(s1, s2));
We can’t use strings with conditional statements in the same way you do numbers in solidity i.e. you can’t compare them with == or you’ll get an error:
TypeError: Built-in binary operator == cannot be applied to types string memory and string memory.
A work around is to hash the string first like this
function add(string memory _x, string memory _y) public returns(bool) {
return keccak256(abi.encodePacked(_x)) == keccak256(abi.encodePacked(_y));
}
I hope this has served as a good introduction to using strings in Solidity and demystified using smart contracts to store text.
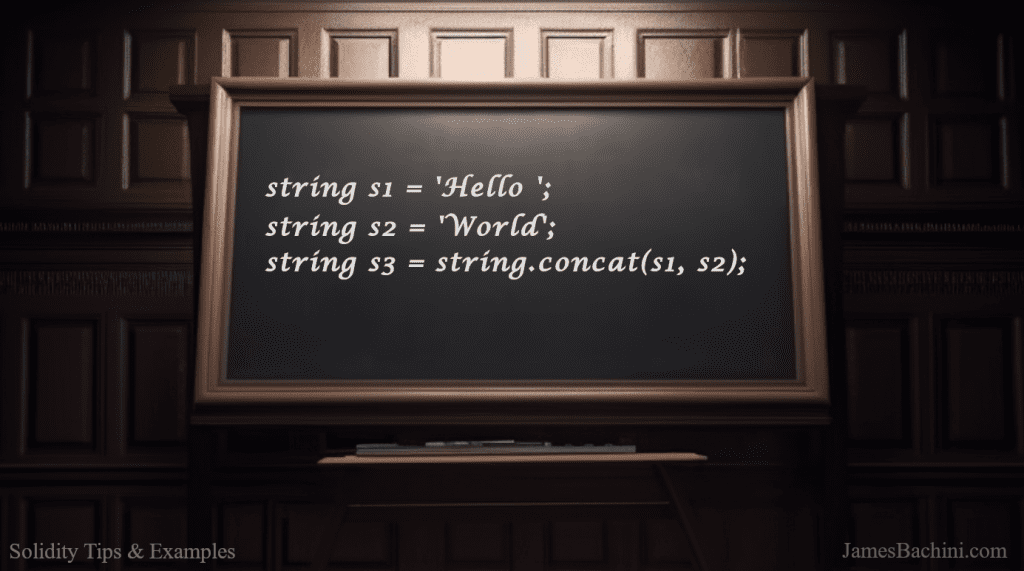