A struct in Solidity is a data type that allows you to group together multiple variables of different data types into a single unit. This is useful because it gives us a convenient container to package structured data, it enables us to pack variables into memory slots more efficiently and it allows us to get around the 16 variable limit which leads to “stack too deep” errrors.
Structs In Solidity Example Code
To define a struct in Solidity, you use the struct keyword followed by the name of the struct, and then list the variables that make up the struct inside curly braces
struct Newb {
string name;
uint age;
address addy;
}
Once you've defined a struct, you can create instances of it by declaring a variable of the struct type and initializing its fields
Newb james = Newb("James", 21, 0x0);
We can also create an array of structs or a mapping of ids or address to struct data.
Newb[] public newbies; // an array
mapping (address => Newb) public newbs; // a mapping
We can then push a value into the newbies array like so:
function addMe public () {
newbies.push(Newb('James',21,msg.sender);
}
And to access that data we can simply add the value key onto the end of the variable i.e.
uint realAge = newbies[0].age;
There is a full example of how to use structs in the Solidity Snippets code repository on Github: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Structs.sol
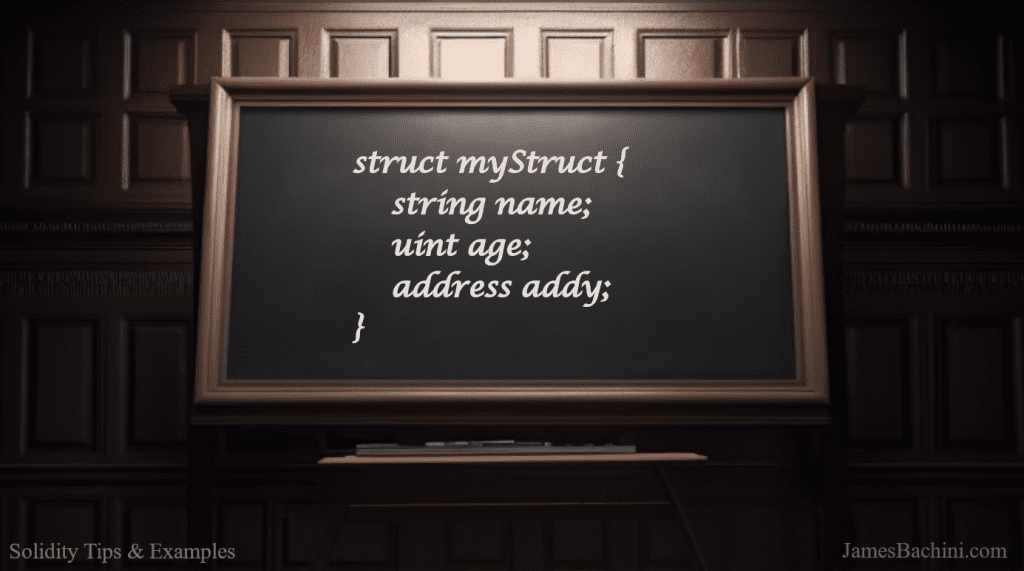
Packing Structs To Optimize Gas
Packing structs in Solidity can be an effective way to optimize gas costs by reducing the number of storage reads and writes needed to access multiple variables. It involves using smaller data types and ordering the variables within the struct in a way that maximizes space utilization. However, it’s important to balance the potential gas savings with the impact on code clarity and maintainability.
It’s important to note that the order of the variables within the struct also matters. When you pack smaller types next to each other, you want to order them in a way that maximizes the space utilization. For example, if you have a struct with two uint64 variables and a bool variable, you would want to order them as follows:
struct MyStruct {
uint64 var1;
bool var2;
uint64 var3;
}
By placing the bool variable in between the two uint64 variables, you are able to pack all three variables within two 256-bit storage slots rather than three.
Packing structs can come with a trade-off in terms of readability and maintainability of the code. It can make the code more complex and harder to understand. Always weigh potential gas savings against the impact on code clarity.