Variables in Solidity can be classified into several categories based on their data types and scope. In this article we will explore all the different uses of variables in Solidity and look at some example code.
- Variables in Solidity
- Variable Scope In Solidity
- Solidity Data Types
- Solidity Variables Examples
- Conclusion
Variables in Solidity
Solidity variables are used to store and manipulate data in our smart contracts. They come in a variety of types, including integers, booleans, addresses, bytes, strings, and arrays.
Each data type has its own characteristics and usage patterns, and understanding how to use them effectively is essential for writing efficient smart contracts.
The two key concepts to understand is the variable scope which defines the access to variables and the different types of variables in Solidity, including their syntax, usage, and limitations. By the end of this article, you should have a good understanding of how to use Solidity variables, and be able to implement them effectively in your own smart contracts.
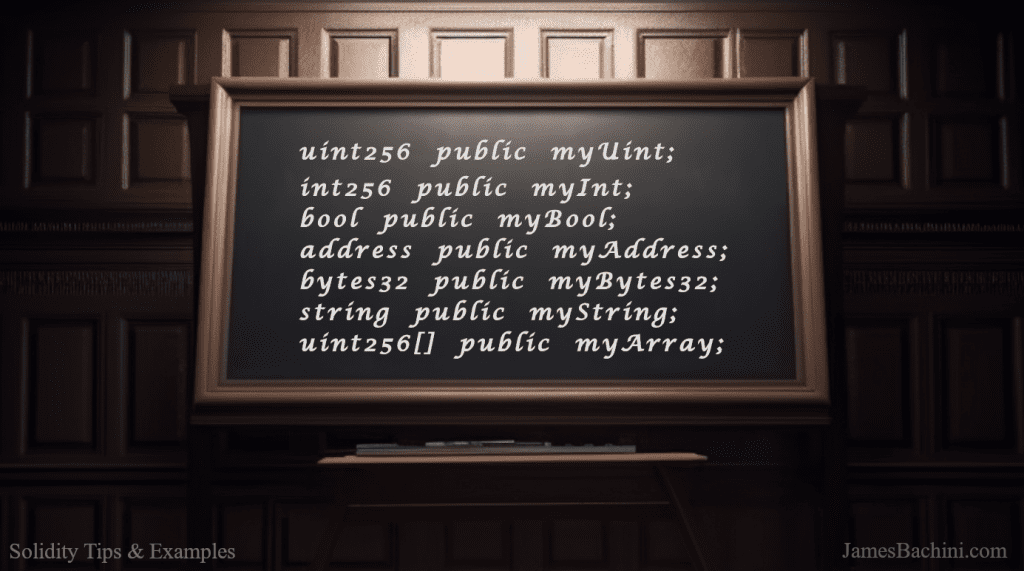
Variable Scope In Solidity
Variable scope refers to the area of a contract where a variable is accessible and can be used. In Solidity variables can be defined within a certain context or area, such as a function or a block of code. The scope of a variable determines where it can be accessed and used within the smart contract.
- State Variables – These are variables that are declared at the contract level and are stored permanently on the blockchain. They hold the contract’s data, and their values can be read and modified by any function within the contract. State variables are declared as
public
,private
, orinternal
when definedpublic
: A public state variable can be accessed from any other contract or externally through a getter function. A getter function with the same name as the public variable is automatically generated by Solidity, which allows external contracts to read the variable’s value.private
: A private state variable can only be accessed from within the contract that defines it. This means that other contracts or external accounts cannot read or modify the variable’s value. This isn’t truly private as all on-chain data is transparent and available via a block explorer.internal
: An internal state variable can be accessed from within the contract that defines it, as well as from any contract that inherits from that contract. This means that any derived contract can read or modify the value of an internal state variable.
- Local Variables – These are variables that are declared inside a function or a block and are only accessible within that function or block. They are not stored on the blockchain and are discarded once the function or block completes execution
- Global Variables – These are variables that are predefined by the EVM (Ethereum virtual machine) and are accessible from any part of the contract. Examples of global variables include
msg.sender
,block.timestamp
, andblock.number
Solidity Data Types
Solidity is a strongly typed programming language in which every variable must be explicitly declared with a specific data type, and the type of a variable cannot be changed after it has been declared. This means that the type of a variable is checked at compile time, rather than at runtime, which helps to prevent errors and make the code more robust.
In a strongly typed programming language, each data type is associated with a set of operations that can be performed on that type. For example, you can add two integers, but you cannot add an integer and a string. If you try to perform an operation that is not valid for a particular data type, the compiler will generate an error.
Solidity has various data types that are used to store different types of values which we will go through:
Boolean
The Boolean data type is used to represent true or false values. In Solidity, the Boolean data type is declared using the keyword “bool”. It can have only two values, either true or false.
bool isTrue = true;
bool isFalse = false;
if (isTrue == true) // doSomething;
Integer
The Integer data type is used to represent whole numbers. In Solidity, there are different integer data types based on the number of bits used to store the value. For example, uint8 is an 8-bit unsigned integer, and int256 is a 256-bit signed integer.
The most common type of integer used in smart contracts is uint256 (also defined shorthand as just uint). This is an efficient way to store large numbers as it fits comfortably into a 32 byte standard memory slot.
uint myNumber = 99999999999;
uint8 mySmallNumber = 26;
Address
The Address data type is used to store Ethereum addresses. In Solidity, an address is a 20-byte value. It is declared using the keyword “address”.
Addresses can be used to define either a users wallet address or a contract address.
address vitalik = 0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045;
Array
The Array data type is used to store a collection of values of the same type. In Solidity arrays can be of fixed or dynamic size. A fixed-size array is declared using the syntax “type[size]”, while a dynamic-size array is declared using the syntax “type[]”.
Nested arrays are arrays that contain other arrays as elements. This means that each element in the outer array is itself an array.
uint[4] fixedArray = [1,2,3,4];
string[] dynamicArray;
string.push('test');
uint[][] nestedArray;
nestedArray[0][0] = 1;
Struct
The Struct data type is used to create custom data types that can contain multiple values of different types. In Solidity, a struct is declared using the keyword “struct”.
struct User {
string username;
uint128 age;
uint128 foobar;
}
By using two uint128 unsigned integers in the struct above it has been possible to pack 3 variables in to two memory slots.
Enum
I honestly don’t remember ever using or seeing a enum variable in Solidity but it’s available. The Enum data type is used to define a set of named constants. In Solidity, an enum is declared using the keyword “enum”.
enum Status { Pending, Approved, Rejected }
Status status1 = Status.Pending;
Solidity Variables Examples
The full code for this example is available at: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Variables.sol
In this contract, we define several state variables of different types, including uint256
, int256
, bool
, address
, bytes32
, string
, and uint256[]
(an array of uint256
values).
We also define an enum State
with two possible values (INACTIVE
and ACTIVE
) and a struct Person
with two fields (username
and age
).
In the constructor, we initialize the state variables with some initial values.
We also define three functions that allow us to modify the state variables:
setUint
allows us to set the value ofmyUint
.setPerson
allows us to set the value ofmyPerson
.addToArray
allows us to add an element to the end ofmyArray
.
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
contract Variables {
uint256 public myUint;
int256 public myInt;
bool public myBool;
address public myAddress;
bytes32 public myBytes32;
string public myString;
uint256[] public myArray;
enum State { INACTIVE, ACTIVE }
State public myState = State.INACTIVE;
struct Person {
string username;
uint256 age;
}
Person public myPerson;
constructor() {
myUint = 123;
myInt = -456;
myBool = true;
myAddress = msg.sender;
myBytes32 = 0x0;
myString = "Hello, world!";
myArray = [1, 2, 3, 4, 5];
myPerson = Person("Alice", 30);
}
function setUint(uint256 _newValue) public {
myUint = _newValue;
}
function setPerson(string memory _username, uint256 _age) public {
myPerson = Person(_username, _age);
}
function addToArray(uint256 _newValue) public {
myArray.push(_newValue);
}
}
Conclusion
By understanding the different types of variables, their usage patterns, and the visibility specifiers available in Solidity, developers can create efficient, secure, and effective smart contracts.
The ability to store data on a decentralized network in trustless smart contracts is a new and novel technology. It allows us to create permissionless applications which rely on no 3rd parties. Understanding the flow of data through Solidity variables is a key step towards mastering this exciting opportunity.