ERC1155 offers a more flexible and efficient way of managing fungible and non-fungible tokens, making it an attractive option for developers who want to create complex digital assets with multiple use cases.
In this blog post, we’ll dive into the details of ERC1155 and explore why it’s becoming a preferred choice for Solidity developers looking to create innovative blockchain-based applications.
- Why Use ERC1155 Token
- Simple ERC1155 Token Contract
- ERC1155 OpenZeppelin Library
- NFT JSON Data
- ERC1155 Token Functions
- Conclusion
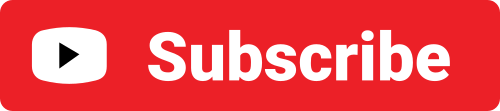
Why Use ERC1155 Token
One of the distinctive features of ERC1155 is that it enables a single smart contract to represent multiple tokens at once, allowing for a more flexible and efficient management of both fungible and non-fungible tokens.
This means that instead of deploying a new contract for each token type, a single ERC1155 token contract can hold the entire system state, resulting in significant gas savings, reduced deployment costs, and complexity.
ERC1155 also provides batch operations that allow developers to operate over multiple tokens in a single transaction efficiently. These features make ERC1155 a more versatile and powerful token standard that can handle complex digital assets with multiple use cases, making it an attractive option for developers looking to build innovative blockchain-based applications.
There is more information on ERC1155 vs ERC721 here: https://jamesbachini.com/erc721-vs-erc1155/
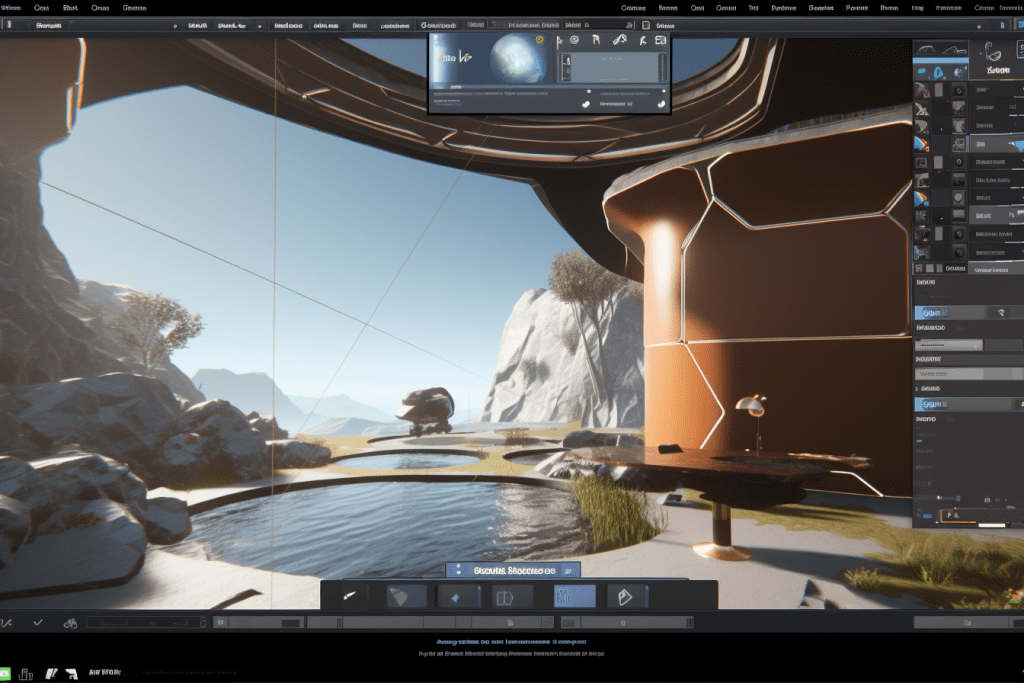
Simple ERC1155 Token Contract
The full code for this contract is available in the Solidity Snippets Github Repository: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/ERC1155.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
contract MyNFT is ERC1155 {
uint256 public constant gameItem1 = 1;
uint256 public constant gameItem2 = 2;
uint256 public constant gameItem3 = 3;
constructor() ERC1155("https://ethereumhacker.com/nfts/{id}.json") {
_mint(msg.sender, gameItem1, 1, "");
_mint(msg.sender, gameItem2, 1, "");
_mint(msg.sender, gameItem3, 100, "");
}
}
ERC1155 OpenZeppelin Library
The code above relies heavily on the OpenZeppelin library where all the token functions are defined. It’s a good idea to have a read through this contract to get a feel for how the code works. Note that ERC1155 is a little more abstract and complex than ERC721.
There are a number of extensions in the framework too
- ERC1155Burnable.sol – This extension adds the ability to burn ERC1155 tokens, allowing for a more flexible management of token supply. It includes a function that allows token holders to burn a specific amount of tokens, reducing the total supply of the token.
- ERC1155Pausable.sol – This extension adds the ability to pause and unpause the transfer and minting functions of the ERC1155 token. This is useful in situations where the token needs to be temporarily frozen, such as during a security audit or when there is a bug in the contract that needs to be fixed.
- ERC1155Supply.sol – This extension adds a total supply variable to the ERC1155 token, allowing developers to track the total supply of each token type. It also includes functions to increase or decrease the supply of a specific token type, providing a more flexible management of token supply.
- ERC1155URIStorage.sol – This extension adds storage for token URI data to the ERC1155 token contract, allowing developers to store and retrieve metadata associated with each token. This metadata can include information about the token’s name, symbol, image, and other properties.
- IERC1155MetadataURI.sol – This is an interface that defines a function to retrieve the metadata URI associated with a specific ERC1155 token. It’s used in conjunction with the ERC1155URIStorage extension to provide a standardized way of retrieving metadata associated with ERC1155 tokens.
NFT JSON Data
JSON {"JavaScript": "Object Notation"}
is a lightweight data format that is easy for humans to read and write, and easy for machines to parse and generate. It is commonly used for data formatting in API’s and is also used to store metadata for NFTs.
Metadata can include information about the creator, title, description, image, video, audio, and other attributes of the token. This metadata is important because it provides additional context and information about the NFT, which can help potential buyers to understand its value and uniqueness.
Opensea has its own standard for formatting JSON metadata associated with each NFT called the Opensea Metadata Standard and it defines a set of required and optional fields that should be included in the JSON metadata for each NFT. Here is an example of the NFT JSON data.
{
"description": "My Cool NFT",
"external_url": "https://jamesbachini.com/misc/1",
"image": "https://jamesbachini.com/misc/nft.jpg",
"name": "James Bachini",
"attributes": [{
"trait_type": "Skillset",
"value": "Useless"
},
{
"trait_type": "Level",
"value": 1
}
]
}
If you are creating multiple NFTs with different traits you can use something like the JANG.js NFT Generator to create the different traits and layer up the images.
Then you’ll need to upload the images and JSON data to IPFS and put a link to the IPFS directory in the NFT smart contract. There are a couple of options for this using 3rd party tools:
The Solidity smart contract needs to return the URI for the correct JSON data when called. To do this we can create a custom tokenURI function:
function tokenURI(uint256 _tokenId) public view returns (string) {
return Strings.strConcat(
baseTokenURI(),
Strings.uint2str(_tokenId)
);
}
Here we are concatenating (adding) the tokenId to the end of the baseTokenURI i.e. tokenId 1 becomes https://jamesbachini.com/misc/1
Once the NFT is minted with the appropriate JSON metadata, it can be listed on Opensea for sale or auction, and potential buyers can view the metadata and image.
ERC1155 Token Functions
Functions
balanceOf(account, id)
This function returns the balance of a specific token id for a given account.
balanceOfBatch(accounts, ids)
This function returns the balances of multiple token ids for multiple accounts.
setApprovalForAll(operator, approved)
This function sets the approval status of an operator for all tokens of a specific account.
isApprovedForAll(account, operator)
This function checks whether an operator is approved for all tokens of a specific account.
safeTransferFrom(from, to, id, amount, data)
This function transfers a specified amount of a specific token from one address to another, and includes optional data that can be used to execute additional functionality on the receiving end.
safeBatchTransferFrom(from, to, ids, amounts, data)
This function transfers multiple tokens of different types and amounts from one address to another, and includes optional data that can be used to execute additional functionality on the receiving end.
supportsInterface(interfaceId)
This function checks whether a contract implements a specific interface.
Events
TransferSingle(operator, from, to, id, value)
This event is emitted when a single token is transferred from one address to another.
TransferBatch(operator, from, to, ids, values)
This event is emitted when multiple tokens of different types and amounts are transferred from one address to another.
ApprovalForAll(account, operator, approved)
This event is emitted when the approval status of an operator for all tokens of a specific account is changed.
URI(value, id)
This event is emitted when the metadata URI of a specific token id is set or updated.
Conclusion
The ERC1155 standard represents a new generation of digital assets. Its distinctive feature of representing multiple tokens in a single smart contract reducing deployment costs. Its support for batch operations and flexible metadata management makes it a powerful tool for developers creating digital asset ecosystems.
As blockchain technology continues to evolve, ERC1155 is poised to become an essential building block for decentralized applications that require multiple tokens to function.