Today we will discuss the differences between ERC721 vs ERC1155 smart contracts and the use cases where each is best suited. These are the two most popular types of smart contracts used for the creation of NFTs (non-fungible tokens).
- ERC721 Smart Contract
- ERC1155 Smart Contract
- Which Should You Use
- ERC721 Example Contract
- ERC1155 Example Contract
- Conclusion
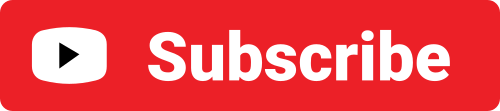
ERC721 Smart Contract
ERC721 is the original standard interface for creating non-fungible tokens (NFTs) on the Ethereum blockchain. These tokens are unique, indivisible, and non-interchangeable. Each token has a unique identifier and can represent a specific asset, such as a piece of artwork or a collectible.
ERC721 smart contracts are used to create NFTs that can be owned, bought, and sold on-chain. The ownership of an ERC721 token is tracked by storing a wallet address in the contract and can be transferred between owners through a secure and transparent process.
ERC721 tokens are often used in profile picture collections, where each token can represent a unique character. They are also popular in the art world, where artists can use them to represent their artwork in a digital form and sell them as digital collectibles.
ERC1155 Smart Contract
ERC1155 is a newer standard interface for creating NFTs on the Ethereum blockchain. Unlike ERC721, ERC-1155 allows for the creation of both fungible and non-fungible tokens within the same smart contract.
Fungible tokens are identical to each other and can be exchanged for one another, such as cryptocurrencies like Bitcoin. Non-fungible tokens, on the other hand, are unique and cannot be exchanged for one another. ERC1155 gives us the option to mint one of a kind or multiples of the same item within a single contract.
_mint(msg.sender, gameItem2, 1, "");
_mint(msg.sender, gameItem3, 100, "");
This makes it more efficient for developers, as they can create multiple tokens within the same contract, reducing gas fees and contract deployment times.
The use cases for ERC1155 tokens are more diverse than ERC721 tokens. They can be used in games, where players can collect both unique and interchangeable items. For example, a game could use an ERC1155 contract to create 100 shields, each with a unique identifier, but all shields are interchangeable in the game.
Which Should You Use
ERC721 is ideal for creating a single series of NFTs, while ERC1155 is better suited for creating a collection of in game items. ERC1155 is more adaptable and expandable but the downside of this is that it is more complex.
If I was going to mint a single NFT or create a set of 10,000 profile picture NFT’s I would use ERC721. If I wanted more complexity to do something like create various in-game items I would use ERC1155.
The choice between ERC721 and ERC1155 ultimately depends on the specific use case and the desired functionality of the token. If you don’t need the additional capabilities of ERC1155 then I would recommend using ERC721 because of it’s elegant simplicity. Devs should consider the features of each standard to determine which is best suited for their project.
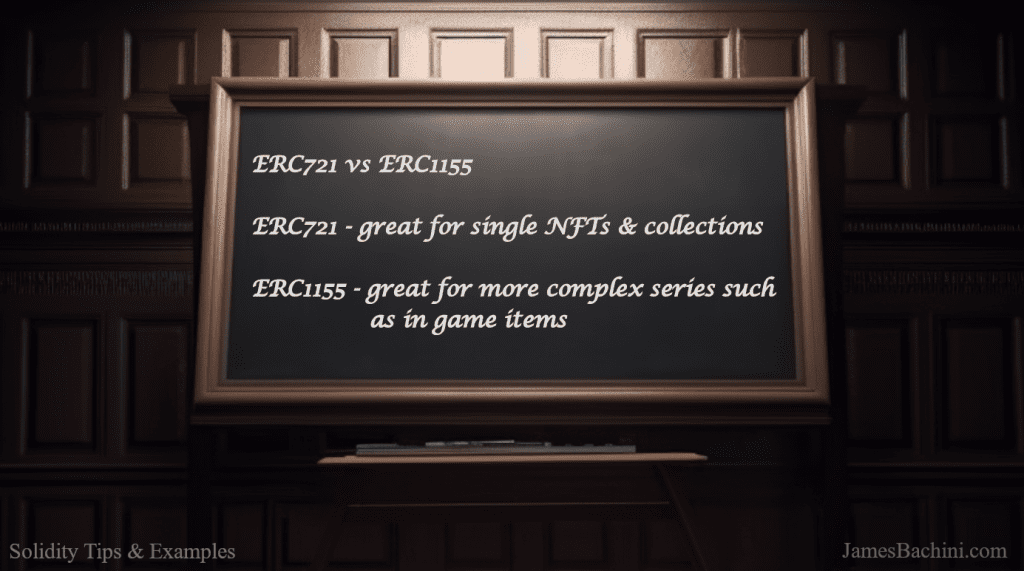
ERC721 Example Contract
Full code available on Github at: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/ERC721.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/utils/Base64.sol";
contract MyNFT is ERC721 {
uint public tokenId;
uint public maxSupply = 1000;
constructor() ERC721("My NFT", "MFT") {
}
function tokenURI(uint) override public pure returns (string memory) {
string memory json = Base64.encode(bytes(string(
abi.encodePacked('{"name": "My NFT", "description": "Whatever", "image": "https://ethereumhacker.com/img/nft.png"}')
)));
return string(abi.encodePacked('data:application/json;base64,', json));
}
function mint() public {
require(tokenId < maxSupply, "All tokens have been minted");
_safeMint(msg.sender, tokenId);
tokenId = tokenId + 1;
}
}
ERC1155 Example Contract
Full code available on Github at: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/ERC1155.sol
pragma solidity >=0.8.0;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
contract MyNFT is ERC1155 {
uint256 public constant gameItem1 = 1;
uint256 public constant gameItem2 = 2;
uint256 public constant gameItem3 = 3;
constructor() ERC1155("https://ethereumhacker.com/nfts/{id}.json") {
_mint(msg.sender, gameItem1, 1, "");
_mint(msg.sender, gameItem2, 1, "");
_mint(msg.sender, gameItem3, 100, "");
}
}
Conclusion
In this article we have looked at the differences between ERC721 vs ERC1155 Solidity token contracts. The takeaway is that ERC1155 adds additional functionality and complexity which may or may not be useful depending on what you are doing.
If you need a contract to create multiple different tokens with different quantities and a mix of fungible vs non-fungible items then ERC1155 is the way to go.
If you want a simpler contract to just mint a single NFT or collection then ERC721 is elegant and will do what you need it to do.
With the continued growth of the NFT market and the Ethereum ecosystem, both standards will likely continue to play an important role in the creation and exchange of digital assets and the future of NFTs.