In this tutorial we will be setting up a Rust script to connect to a smart contract on a blockchain network to display on-chain data using the ethers-rs library.
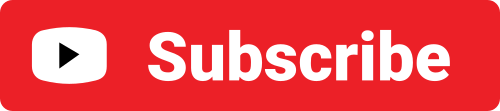
Let’s start by setting up a new Rust project (you’ll need rust installed on your device and I’ll be using Windows subsystem for linux which is recommended).
cargo new ethers-test
Add the following to your dependencies in the Cargo.toml file
[dependencies]
ethers = "2.0"
tokio = { version = "1", features = ["rt-multi-thread", "macros"] }
We will also need an Infura API key. The infura key enables us to connect to one of their nodes which is connected to the p2p Ethereum network. Let’s export this to an environmental variable
export INFURA_API_KEY=your_infura_api_key_here
We then need a contract address and ABI (application binary interface) for the smart contract we want to interact with. I’m going to be using this contract on Goerli testnet: 0xFBA3912Ca04dd458c843e2EE08967fC04f3579c2
We can put the contract address in to https://goerli.etherscan.io and download the contract ABI. https://goerli.etherscan.io/address/0xfba3912ca04dd458c843e2ee08967fc04f3579c2#code
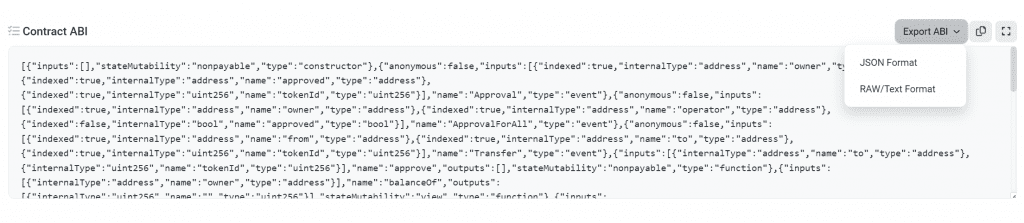
Let’s save the download to the following location src/abi.json
Now let’s go in and edit the src/main.rs file. The code for this can also be found on Github here:
use ethers::prelude::*;
use ethers::providers::{Provider, Http};
use std::sync::Arc;
use std::env;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let contract_address = "0xfba3912ca04dd458c843e2ee08967fc04f3579c2".parse::<Address>()?;
abigen!(IERC721, "./src/abi.json");
let rpc_url = format!("https://goerli.infura.io/v3/{}", env::var("INFURA_API_KEY")?);
let provider = Provider::<Http>::try_from(rpc_url.as_str())?;
let provider = Arc::new(provider);
let contract = IERC721::new(contract_address, provider.clone());
let function_name = "symbol";
let function_params = ();
let result: String = contract.method(function_name, function_params)?.call().await?;
println!("{}", result);
Ok(())
}
Now let’s build and run the code
cargo run
And you should see an output of “WAGMI” which is the data returned from the smart contract.
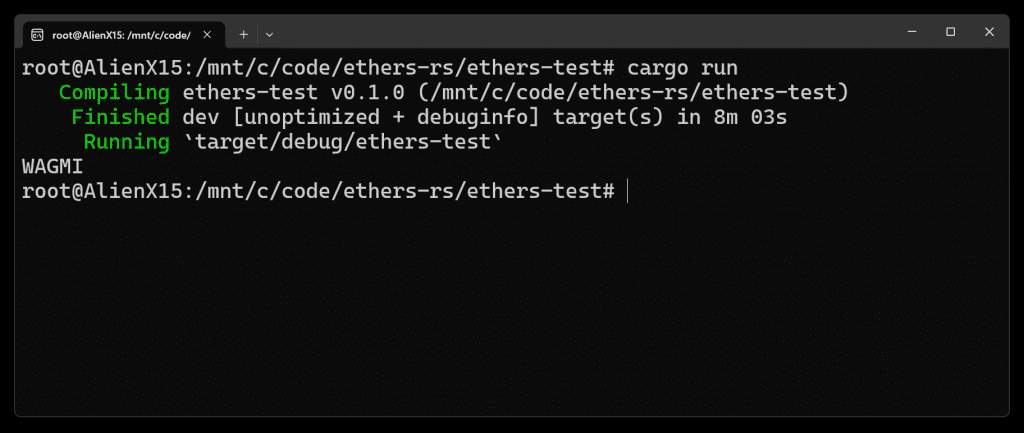