I find it surprising and rare when using new technology and it just works and is intuitive. Getting up to speed with Etherspot and account abstraction is one of those rare occasions. In this tutorial I’ll show you how I created a little demo to create a smart wallet, fund it and then send batched transactions using the bundler.
How Does Etherspot Work
Account abstraction is the concept of moving wallet addresses away from user accounts where we are signing transactions in Metamask to using smart wallets which are contracts with additional functionality to give a better user experience.
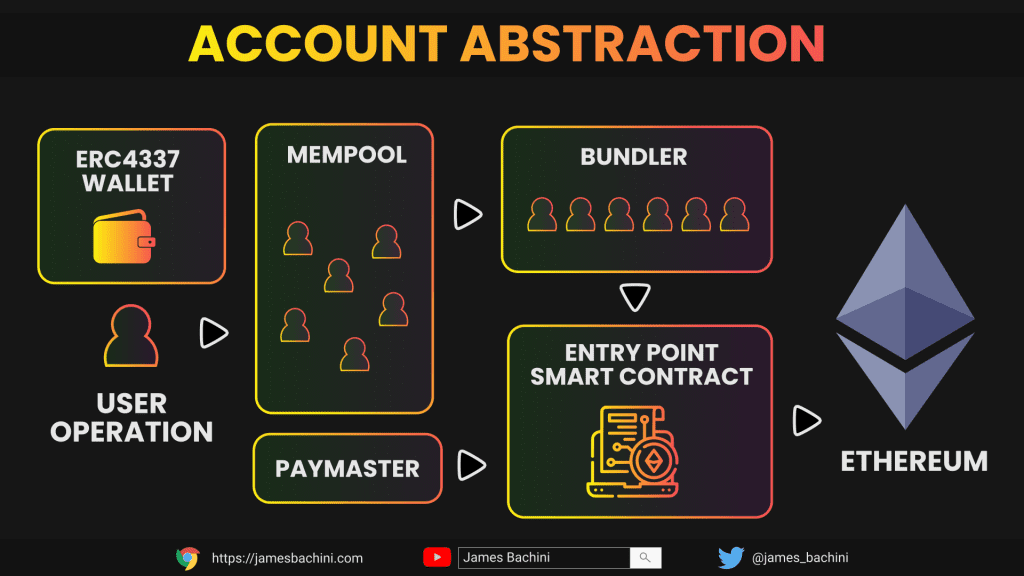
Account abstraction smart wallets can bundle transactions, have gas free sponsored transactions, social recovery mechanisms etc. In essence we can create user interfaces without the need to connect up a external wallet to sign a transaction every time we want to write data.
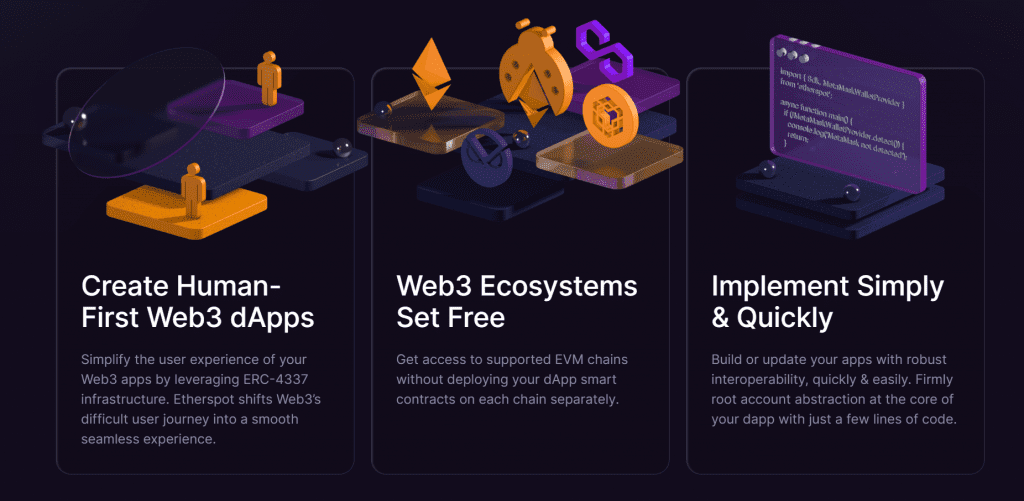
It’s worth mentioning that there is a trade off here where we are using a centralized service to provide this improved experience. A bundler could be censored and provide a single point of failure for a decentralized application.
That being said it’s a trade off that many in the Ethereum ecosystem feel is worthwhile and valuable. For web3 to reach the masses it needs to become easier to use and more secure for non-crypto-native users.
We are going to set up a react frontend with the Etherspot SDK, then create a wallet address on Goerli testnet, send funds and then set up a bundled transaction using the SDK execute.
You can try out the dApp we are about to build here: https://jamesbachini.github.io/Etherspot-Account-Abstraction/
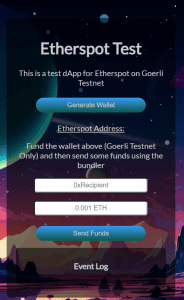
Setting Up React
You’ll need NodeJS and create-react-app installed on your system. Then go ahead and run the following commands
npx create-react-app etherspot-test
cd etherspot-test
npm i -s ethers
npm i -s @etherspot/prime-sdk
npm run start
This should create a local development server and open up a web page with a simple react app.
We can then go into the src directory and open up the App.js file to create our account abstraction dApp.
The full source code can be found here: https://github.com/jamesbachini/Etherspot-Account-Abstraction/blob/main/frontend/src/App.js
Creating A Wallet
The first code we are going to look at is creating a smart wallet.
const generateWallet = async () => {
const randomWallet = ethers.Wallet.createRandom();
setPrivateKey(randomWallet.privateKey);
primeSdk = new PrimeSdk({ privateKey: randomWallet.privateKey}, { chainId: 5, projectKey: '' })
const address = await primeSdk.getCounterFactualAddress();
setEtherspotWalletAddress(address);
setEventLog(`Generated new address: ${address}`);
}
Here we are first creating a random wallet address using ethers.js and then using the privateKey from this to generate a Etherspot Prime smart wallet.
We can then fund this wallet by sending some Goerli ETH to the wallet address.
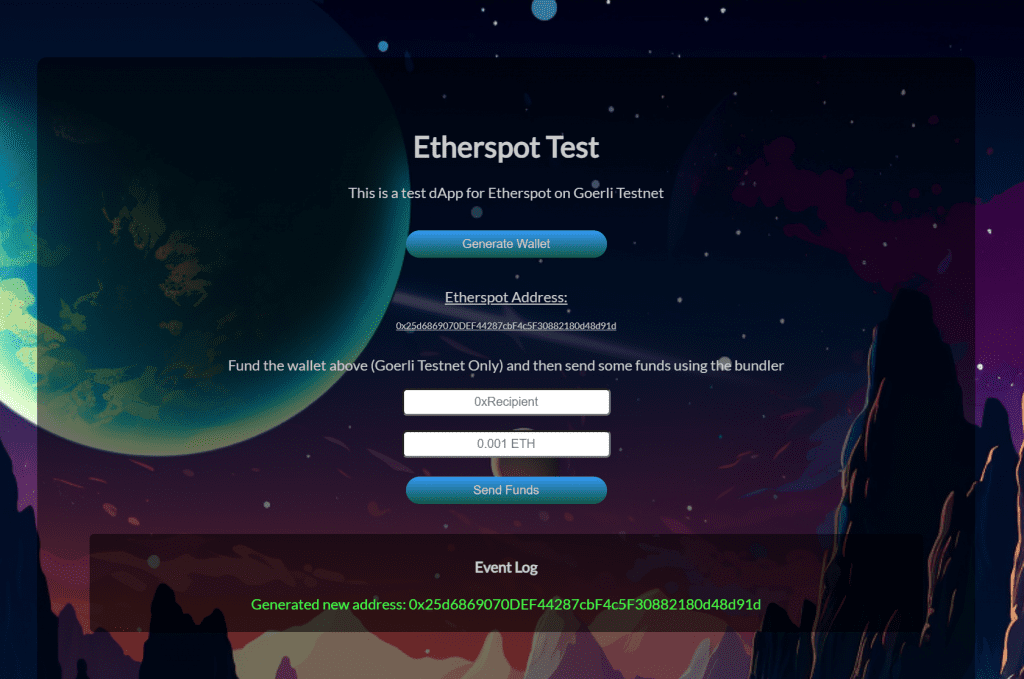
Sending Funds
The next bit of code that I want to look at is the sending of funds. This creates a bundled transaction. We can actually add more than just a single tx to this before sending and it will all be sent together.
const sendFunds = async () => {
const toAddress = document.getElementById('send-address').value;
const stringValue = document.getElementById('send-value').value;
await primeSdk.addUserOpsToBatch({to: toAddress, value: ethers.parseEther(stringValue)});
const op = await primeSdk.estimate();
const uoHash = await primeSdk.send(op);
let userOpsReceipt = null;
const timeout = Date.now() + 300000;
while((userOpsReceipt == null) && (Date.now() < timeout)) {
await new Promise(r => setTimeout(r, 2000));
userOpsReceipt = await primeSdk.getUserOpReceipt(uoHash);
}
}
We start by defining a recipient address and value amount, converting the amount to wei using ethers.parseEther()
This is then passed to primeSdk.addUserOpsToBatch() where we create an estimate and then send the bundle as a userOp.
We finally create a timeout loop while we wait for the transaction to go through as the block is confirmed.
If you look at the transaction in a block explorer you’ll notice it’s coming from the bundler rather than your user wallet or etherspot wallet.
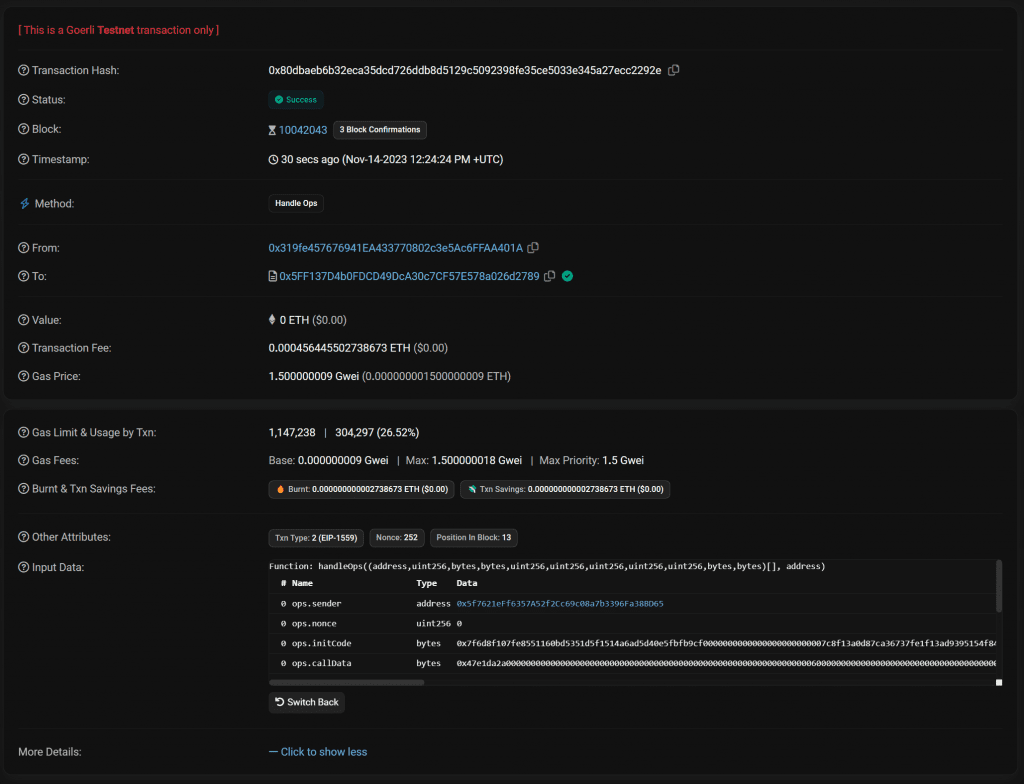
Conclusion
Etherspot provides the best SDK I’ve seen to date for integrating account abstraction into a dApp. There are additional features which you can integrate such as:
- Batching transactions
- Sponsored transactions
- Fiat onramps via Onramper
- Guardians (social recovery)
- Social Logins
- Token Swaps
- Cross chain bridging
It’s an interesting product that I expect I and many other web3 devs will be using in the future. Account abstraction is part of the puzzle that will enable us to obfuscate the complexities of decentralized applications.