Modifiers in Solidity are a way to add a certain condition to functions within a smart contract. They allow developers to define a piece of code that can be reused across multiple functions and contracts, making your code more modular and easier to read.
A modifier is defined using the modifier keyword, followed by a name, and then a block of code enclosed in curly braces. This code block contains conditions that must be met for the modifier to be applied.
Full code available in the Solidity Snippets Github repo: https://github.com/jamesbachini/Solidity-Snippets/blob/main/contracts/Modifier.sol
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.0;
contract Modifier {
modifier onlyVitalik() {
require(msg.sender == 0xd8dA6BF26964aF9D7eEd9e03E53415D37aA96045);
_;
}
function doSomething() external onlyVitalik {
// Only vitalik's wallet can call this function
}
}
In the above example we want to add a modifier condition that checks to see if the msg.sender (person that called the transaction) is Vitalik’s wallet address.
We call this modifier onlyVitalik and we add it to the doSomething() function so that only Vitaliks wallet can access that function.
Modifiers provide a simple, reusable way to add conditional checks before running code. Here are a couple of common examples where they are used are:
- Ownable.sol – the OpenZeppelin library allows you to add the onlyOwner modifier so that only the contract deployer or a set address can access that functionality
- ReentrancyGaurd.sol – another OpenZeppelin library that uses a nonReentrant modifier to prevent reentrancy attacks
I hope this quick breakdown of the modifier implementation within Solidity is of interest and it helps you build out your contracts to become the future of finance… or just a fun NFT collection on testnet.
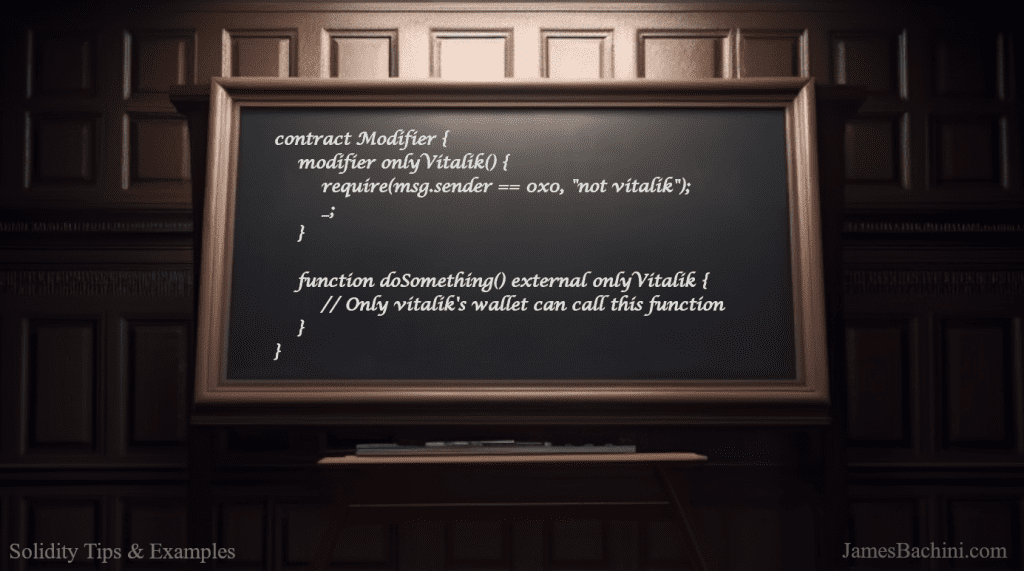