To check a wallet token balance on the Ethereum blockchain using Python you will need the following:
- Infura API Key You can get this by signing up at Infura.
- Python Installed on your device, download here.
- Web3.py This is a Python library for interacting with the Ethereum blockchain.
Once we have our API key ready and Python installed we can install web3.py using the following command
pip install web3
Now let’s create a file called balance.py and add the following code. Note the code is also available in the Github repository: https://github.com/jamesbachini/Web3-Python-Scripts
from web3 import Web3
infura_url = 'https://mainnet.infura.io/v3/YOUR_API_KEY_HERE'
web3 = Web3(Web3.HTTPProvider(infura_url))
wallet_address = '0x123e710c69b6806ef32Cf52e49dCC5EEEc368a22'
token_contract_address = '0x7eae7422f633429EE72BA991Ac293197B80D5976'
token_abi = [
{
"constant": True,
"inputs": [{"name": "owner", "type": "address"}],
"name": "balanceOf",
"outputs": [{"name": "balance", "type": "uint256"}],
"type": "function"
},
{
"constant": True,
"inputs": [],
"name": "decimals",
"outputs": [{"name": "", "type": "uint8"}],
"type": "function"
}
]
token_contract = web3.eth.contract(address=token_contract_address, abi=token_abi)
balance = token_contract.functions.balanceOf(wallet_address).call()
decimals = token_contract.functions.decimals().call()
adjusted_balance = balance / (10 ** decimals)
print(f"Token Balance: {adjusted_balance}")
Enter your API into the URL on line 3 and then let’s run the script with:
python ./balance.py
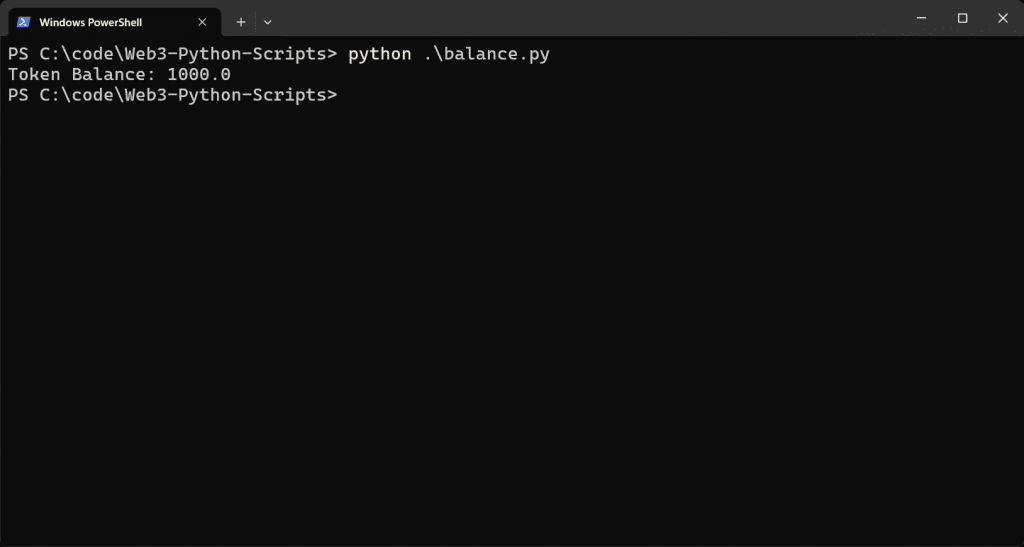
This script will connect to the Ethereum blockchain via Infura, interact with the specified token contract, and print the token balance of the specified wallet address.