A token factory contract is used to deploy tokens from a parent contract within Solidity. In this simple example we will be deploying an ERC20 token from our factory contract.
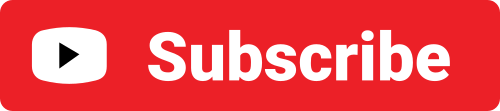
Full source code below and on Github: https://github.com/jamesbachini/Token-Factory-Tutorial
We start with a standard ERC20 token using the OpenZeppelin library. The entire circulating supply of the token is minted to the deployer of the contract via the _mint command in the constructor argument.
The factory contract is a second separate contract which deploys the token. Because the initial mint goes to the factory contract, essentially the contract will own all the tokens, we need to transfer them to the end user that calls the contract.
// SPDX-License-Identifier: MIT
pragma solidity >=0.8.16;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract Token is ERC20 {
constructor(string memory _name, string memory _ticker, uint256 _supply) ERC20(_name, _ticker) {
_mint(msg.sender, _supply);
}
}
contract Factory {
address[] public tokens;
uint256 public tokenCount;
event TokenDeployed(address tokenAddress);
function deployToken(string calldata _name, string calldata _ticker, uint256 _supply) public returns (address) {
Token token = new Token(_name, _ticker, _supply);
token.transfer(msg.sender, _supply);
tokens.push(address(token));
tokenCount += 1;
emit TokenDeployed(address(token));
return address(token);
}
}