You can now use Vyper (a smart contract programming language similar to python) with remix to build and deploy contracts on Ethereum and other EVM blockchains.
The first step is installing the Vyper plugin at https://remix.ethereum.org/ where you’ll find a list of plugins using the icon in the bottom left. You’ll then get an extra tab to compile Vyper code.
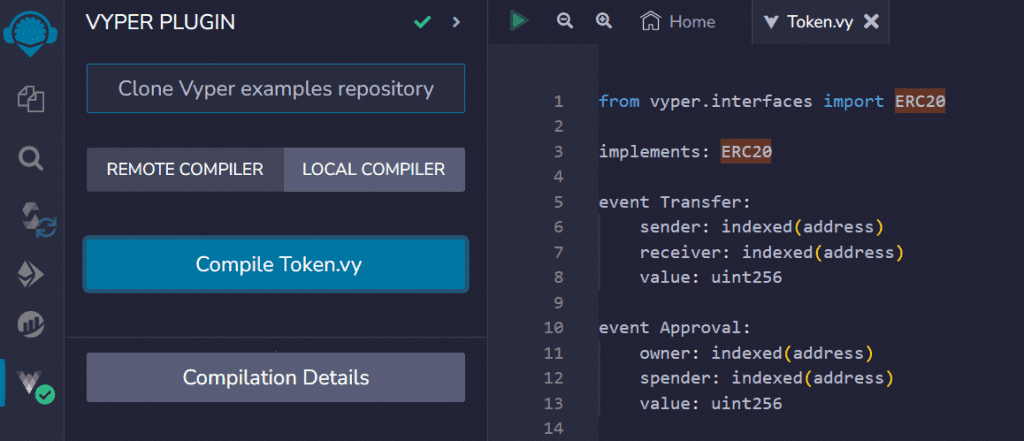
Create a new file and call it something.vy then you can copy and paste in this simplified ERC20 token example.
from vyper.interfaces import ERC20
implements: ERC20
event Transfer:
sender: indexed(address)
receiver: indexed(address)
value: uint256
event Approval:
owner: indexed(address)
spender: indexed(address)
value: uint256
name: public(String[32])
symbol: public(String[32])
decimals: public(uint8)
balanceOf: public(HashMap[address, uint256])
allowance: public(HashMap[address, HashMap[address, uint256]])
totalSupply: public(uint256)
@external
def __init__():
self.name = "vyToken"
self.symbol = "VY"
self.decimals = 18
init_supply: uint256 = 1_000_000 * 10 ** convert(self.decimals, uint256)
self.balanceOf[msg.sender] = init_supply
self.totalSupply = init_supply
log Transfer(empty(address), msg.sender, init_supply)
@external
def transfer(_to : address, _value : uint256) -> bool:
self.balanceOf[msg.sender] -= _value
self.balanceOf[_to] += _value
log Transfer(msg.sender, _to, _value)
return True
@external
def transferFrom(_from : address, _to : address, _value : uint256) -> bool:
self.balanceOf[_from] -= _value
self.balanceOf[_to] += _value
self.allowance[_from][msg.sender] -= _value
log Transfer(_from, _to, _value)
return True
@external
def approve(_spender : address, _value : uint256) -> bool:
self.allowance[msg.sender][_spender] = _value
log Approval(msg.sender, _spender, _value)
return True
Click compile and then you can use the deployment tab just like you would a Solidity smart contract.
You’ll notice the code above pleasantly devoid of curly brackets ❤️
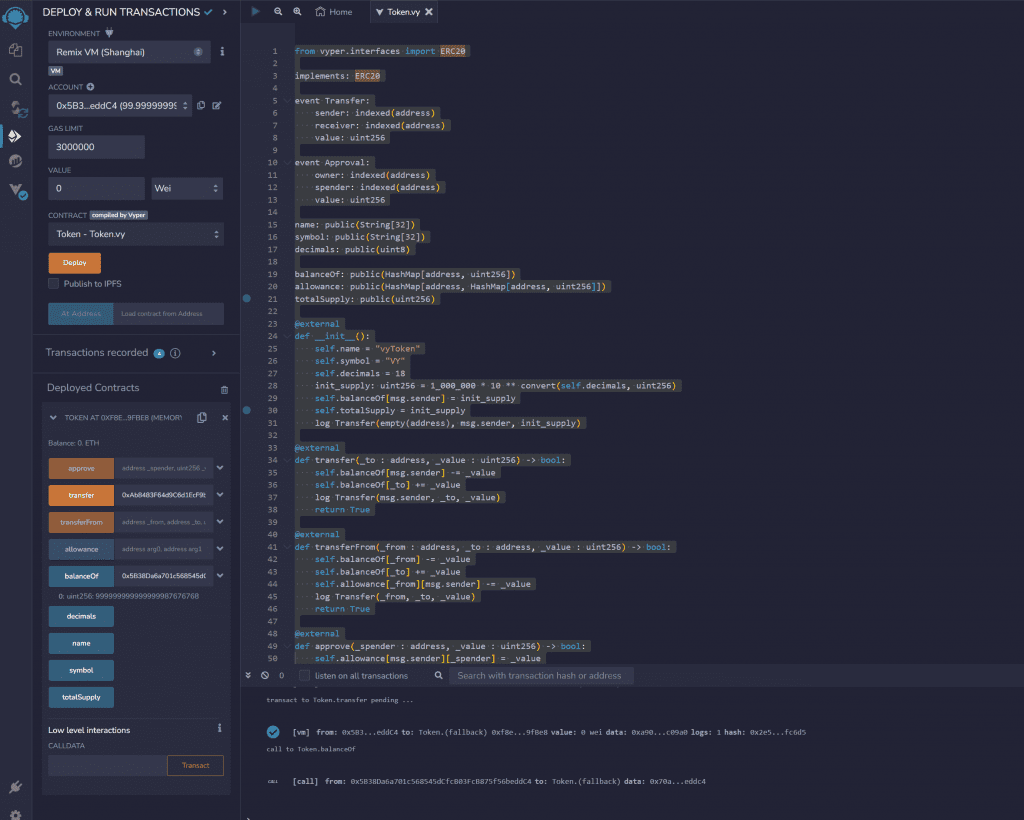