In this article I’ll share how I built a Twitter trading bot to trade a cryptocurrency called DOGE every time Elon Musk tweeted something mentioning it. I’ll be using NodeJS to query the Twitter API and then executing trades on FTX.
- Building A Twitter Trading Bot Video
- The “Elon Effect” Opportunity
- Setting Up API Keys
- Working With Twitter API
- Trade Execution
- Risk Management
- The Code
- Results
Building A Twitter Trading Bot Video
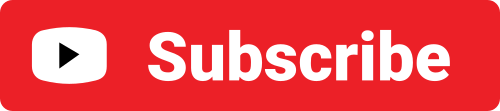
The “Elon Effect” Opportunity
Anything Elon Musk touches turns to gold including zombie coins. Within minutes of each time he tweets about the cryptocurrency DOGE it starts a big upwards move.
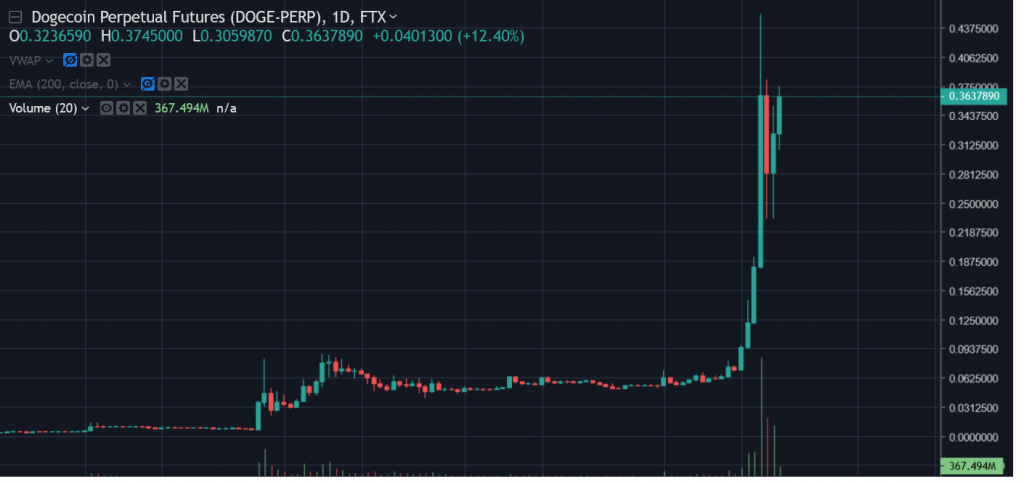
It’s up nearly 100x this year outperforming my entire portfolio and I had zero exposure 😭 I wanted a way to capture some of that upside potential without FOMOing in at the top.
The idea I came up with was to start exploring social trading by monitoring Elon’s twitter account using the Twitter API and then market buy a DOGE perpetual futures contract each time he tweets something. Admittedly I’m not the first person to come up with this simple strategy.
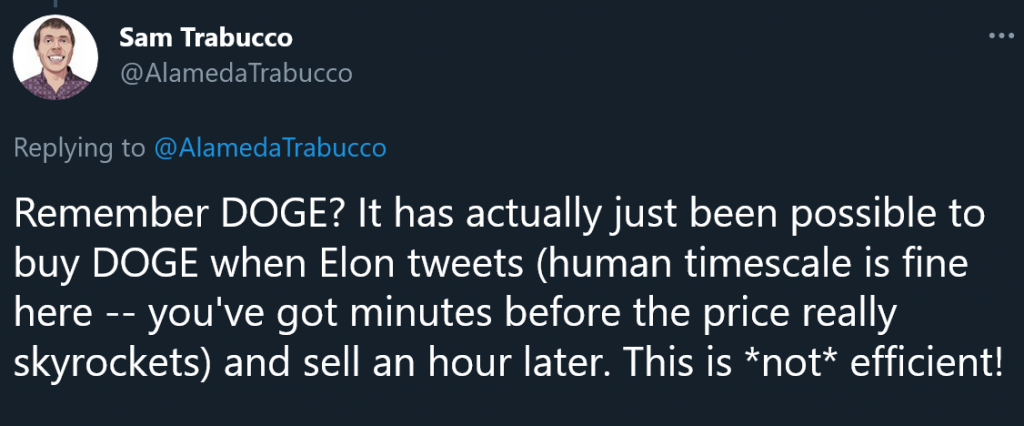
Setting Up API Keys
The first step is to set up API keys for Twitter and a cryptocurrency exchange. I’m going to be using FTX for this demonstration.
Twitter API Keys
If you already have a Twitter account you can head over to https://developer.twitter.com/en/portal/dashboard and set up a developer account and register a new project/app. I already had v1 API keys set up so I used them but v2 should work as well.
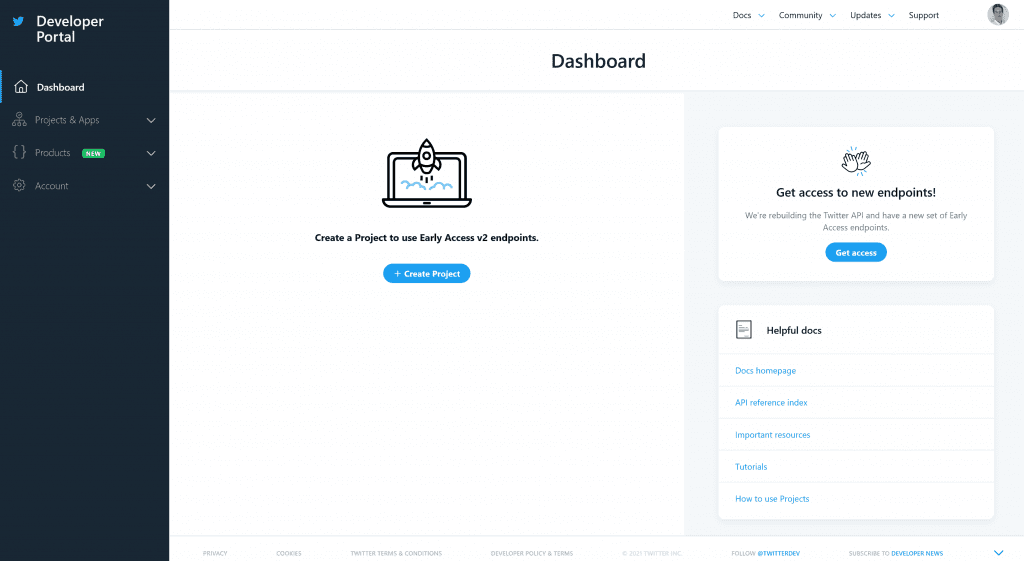
FTX API Keys
I prefer to do this type of trading on FTX rather than Binance because the platform is more set up for active traders in my opinion.
You can set up an account at FTX here: https://ftx.com/#a=StartHere
I set up a sub account to work with as I wanted to use relatively high margin and isolate the risk to a specific amount which was transferred to that sub-account.
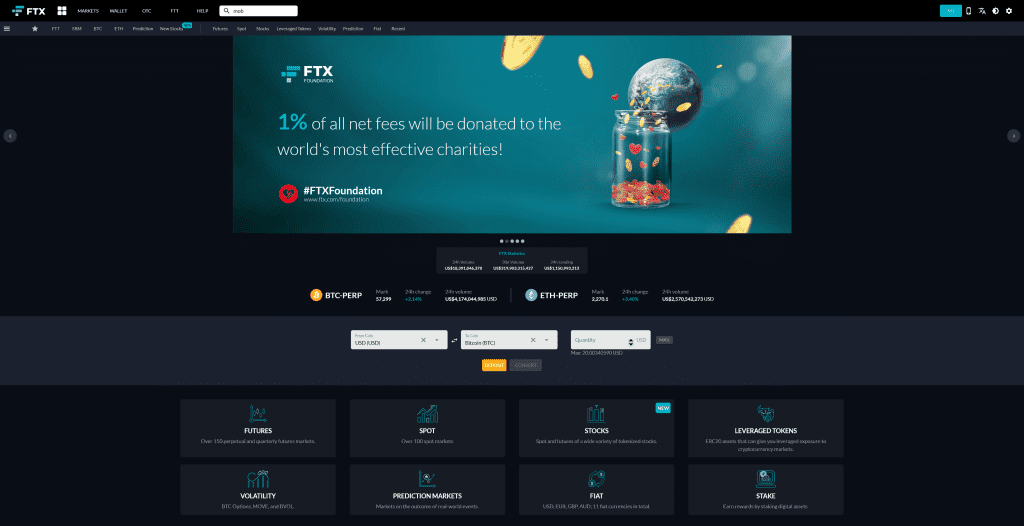
You can set up sub-accounts and API keys from the settings menu.
Working With Twitter API
I used the Twitter NPM module as a base to build on. Before I start going into the code I’d just like to point out that everything is open sourced and the full code is available here: https://github.com/jamesbachini/Twitter-Trading-Bot
So we start by including the module and setting up client credentials
const Twitter = require('twitter');
const config = require('./config.js');
const client = new Twitter({
consumer_key: config.twitterAPI.consumer_key,
consumer_secret: config.twitterAPI.consumer_secret,
access_token_key: config.twitterAPI.access_token_key,
access_token_secret: config.twitterAPI.access_token_secret,
});
The next step is to get the user id of the person we want to monitor, this is a numerical number which can be derived from their username i.e. @elonmusk > 44196397. There are also websites that you can use to find this out manually, just google “twitter user to id”.
const getID = async (username) => {
return new Promise((resolve, reject) => {
client.get('users/lookup', {screen_name: username}, (error, tweets, response) => {
if(error) throw error;
const twitterID = JSON.parse(response.body)[0].id;
resolve(twitterID);
});
});
}
I then wanted to stream live data from his feed as I believe this would be faster than REST based API requests (not tested this assumption).
const filter = { follow: twitterID };
client.stream('statuses/filter', filter, (stream) => {
stream.on('data', (tweet) => {
console.log(tweet.text);
});
});
There’s some quirks here that the tweet.text actually contains a shortened version of the tweet and a bit of error checking was included in the full version. This shows the basics of setting up a stream which will execute instantly upon a new tweet being broadcast.
We can then search through the tweet text for relevant keywords, hash tags and cash tags that we want to execute trades based upon.
Trade Execution
I choose not to use a library or wrapper for the exchange integration and to manually sign transactions. This adds a little more complexity but I think there’s a security benefit to doing so.
Here is the code to execute a simple market order:
const request = require('request');
const crypto = require('crypto');
const ftxOrder = () => {
const ts = new Date().getTime();
const query = {
market: config.market,
side: 'buy',
size: config.quantity,
type: 'market',
price: 0,
}
const queryString = `${ts}POST/api/orders${JSON.stringify(query)}`;
const signature = crypto.createHmac('sha256', config.ftxAPI.apiSecret).update(queryString).digest('hex');
const uri = `https://ftx.com/api/orders`;
const headers = {
"FTX-KEY": config.ftxAPI.apiKey,
"FTX-TS": String(ts),
"FTX-SIGN": signature,
"FTX-SUBACCOUNT": config.ftxAPI.subAccount
};
request({headers,uri,method:'POST',body:query,json:true}, function (err, res, ticket) {
if (err) console.log(err);
if (ticket && ticket.result && ticket.result.id) {
ftxTrailingStop();
} else {
console.log(ticket);
}
});
}
I also wanted to execute a trailing stop loss order which is very similar but uses the following end point and slightly different query parameters.
const uri = `https://ftx.com/api/conditional_orders`;
I tested this manually on it’s own and lost a few dollars to the spread because both orders are market orders which is worth noting.
Risk Management
In my opinion this is one of the rare trades where using high leverage actually makes sense. We are going to be in the trade for a relatively short amount of time to essentially capture that initial burst of interest and get out at the first pull back.
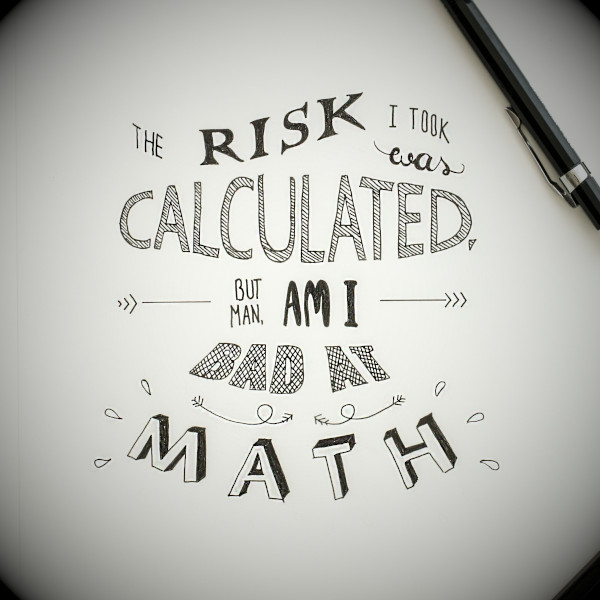
I set the account up to use about 15x leverage which risks the entire balance on each trade. That combined with the lack of sentiment analysis means that if Elon tweets “Doge is over” I’m almost certainly going to lose the entire sub-account.
Having said that from an EV (how to calculate expected value) perspective I think it’s a good bet but… I want to manage my risk as much as possible.
The first step is to set up a sub account. This isolates the position from wiping out the entire account balance. It’s also very useful for testing as I can just put a small amount in account and if there are any bugs in my code it can’t go nuts and spend all my funds on a huge leveraged DOGE position.
The second step was to create an exit plan. I decided to use a trailing stop loss as this would limit the initial risk and then also provide a dynamic take profit level as the price moves up. I’d expect high volatility so it needs some wiggle room but too much and the account will get liquidated before the stop loss triggers if things go south from the start.
Probably over time I will add more complex code to scale into a position over a 60 second period and increase the stop loss size as price moves up so I can limit downside risk but let the profits run.
The Code
The twitter trading bot source code is here:
https://github.com/jamesbachini/Twitter-Trading-Bot
If you want to run this you’ll need to install nodeJS on your device or ideally on a server.
There’s an example configuration file which provides the basic setup. Edit the fields and rename config-example.js to config.js
I’d highly recommend this code isn’t run for anything other than testing as it’s not production ready. There will be bugs and quirks and badly handled exchange response issues which can and will cost money if you try to use this in production.
I made it available for education purposes as a entry point for people to start using this type of script for social trading.
If anyone wants to add to the code, refactor it, test it, make it less like something that was put together in an afternoon then feel free via a pull request.
Disclaimer
This twitter trading bot is for demonstration purposes only. It was put together in a couple of hours and is not fully tested or production ready code. Use it for educational purposes only and build something better if you want to use it with real funds.
Do not use this script with your live trading account, and if you ignore this and lose funds do not blame me
Results
I ran the twitter trading bot from the 16th April to 19th April while DOGE was the 3rd most traded cryptocurrency on FTX and all the time Elon didn’t say a word about it. I’m in half a mind that his lack of tweeting about it is a signal in itself and perhaps he’s buying some up.
I decided to leave that test running and expand it out on another sub account to bid on crypto cash tag mentions from popular crypto influencers. I started with a $20 budget and ran it for 24 hours. The first night I had an issue where it was only tracking a couple of the influencers. This was caused by a bug in the way the Twitter API uses a numerical ID and then a user.id_str string which is a totally different number. This morning I fired it up for a few hours once the bug was fixed before I posted this article out.
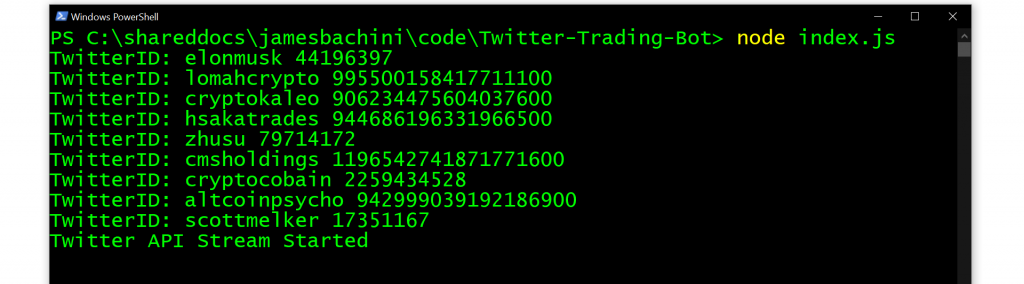
It executed a trade and manually closed it out about half hour later for a profit of $0.14. I’d say the influencer’s mention didn’t affect the price of the asset significantly in this test.
There is a curve to the effectiveness of this strategy. The more engagement an influencer has the better and the lower the market cap of the asset the better. A smaller influencer talking about Bitcoin isn’t going to move the price. For that reason I’ll be running this strategy in future for specific opportunities where there is significant potential market impact.
The code still isn’t production ready but it is open source and available to use as a starting point if anyone wants to use it for a more advanced social trading application.
The next step will be to look at Uniswap’s new v3 code and get ready for the next big alt season which I think will be happening once Ethereum’s optimism layer two solution is rolled out and everyone starts searching for “Uniswap gems” again. In that kind of environment when low cap, low liquidity altcoins are being speculated on and shilled by influencers this type of script could do really well.
Roll on summer of DeFi.